C++怎么实现多线程并行处理
时间: 2024-04-18 14:06:29 浏览: 124
C++中可以使用以下几种方式实现多线程并行处理:
1. 使用标准库中的`std::thread`创建一个新的线程,并将需要执行的函数作为参数传入。例如:
```c++
#include <iostream>
#include <thread>
void myFunction(int arg1, int arg2) {
std::cout << "Thread ID: " << std::this_thread::get_id() << std::endl;
std::cout << "Arg1: " << arg1 << ", Arg2: " << arg2 << std::endl;
}
int main() {
std::thread t(myFunction, 10, 20);
t.join();
return 0;
}
```
2. 使用OpenMP库来实现并行化。OpenMP是一个面向共享内存的并行编程API,它可以将指定的代码块并行化执行。例如:
```c++
#include <iostream>
#include <omp.h>
int main() {
#pragma omp parallel num_threads(4)
{
std::cout << "Hello World from thread " << omp_get_thread_num() << std::endl;
}
return 0;
}
```
3. 使用C++11中的`std::async`和`std::future`来实现异步执行。`std::async`可以异步地执行一个函数,并返回一个`std::future`对象,可以使用`std::future::get()`方法来获取函数的返回值。例如:
```c++
#include <iostream>
#include <future>
int myFunction(int arg1, int arg2) {
return arg1 + arg2;
}
int main() {
std::future<int> result = std::async(std::launch::async, myFunction, 10, 20);
std::cout << "Result: " << result.get() << std::endl;
return 0;
}
```
以上是几种常用的C++实现多线程并行处理的方法。需要注意的是,在多线程编程中,需要避免数据竞争和死锁等问题,这些问题需要通过合适的同步机制来解决。
阅读全文
相关推荐

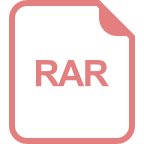





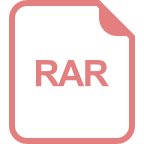






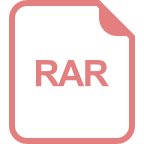
