利用css、JQ和HTML设计一个B/S网页登录页面
时间: 2024-01-16 12:02:30 浏览: 26
好的,让我给您讲一下如何利用 HTML、CSS 和 jQuery 设计一个 B/S 网页登录页面。
首先,在 HTML 文件中添加一个表单用于输入用户名和密码:
```html
<form>
<div>
<label for="username">用户名:</label>
<input type="text" id="username" name="username">
</div>
<div>
<label for="password">密码:</label>
<input type="password" id="password" name="password">
</div>
<div>
<button type="submit" id="submit-btn">登录</button>
</div>
</form>
```
接下来,在 CSS 文件中为表单添加样式,使其呈现出美观的外观:
```css
form {
width: 300px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
box-shadow: 0 0 10px #ccc;
}
label {
display: inline-block;
width: 80px;
text-align: right;
margin-right: 10px;
}
input[type="text"],
input[type="password"] {
width: 200px;
padding: 5px;
border: 1px solid #ccc;
border-radius: 3px;
outline: none;
}
button {
margin-top: 10px;
padding: 5px 10px;
border: none;
border-radius: 3px;
background-color: #007bff;
color: #fff;
cursor: pointer;
}
```
最后,在 JavaScript 文件中使用 jQuery 监听表单提交事件,获取表单输入的用户名和密码,然后发送 AJAX 请求到后端验证用户信息,最后根据验证结果做出相应的处理:
```javascript
$(function() {
$("form").submit(function(event) {
// 阻止表单默认提交行为
event.preventDefault();
// 获取用户名和密码
var username = $("#username").val();
var password = $("#password").val();
// 发送 AJAX 请求到后端验证用户信息
$.ajax({
url: "/login",
method: "POST",
data: { username: username, password: password },
success: function(data) {
// 根据验证结果做出相应的处理
if (data.success) {
alert("登录成功!");
// 跳转到首页
window.location.href = "/";
} else {
alert("用户名或密码错误,请重新输入!");
// 清空密码输入框
$("#password").val("");
}
},
error: function() {
alert("网络错误,请稍后再试!");
}
});
});
});
```
这样,一个简单的 B/S 网页登录页面就设计完成了。需要注意的是,上述代码仅为示例,实际开发中还需要对用户输入进行一些安全性检查。
相关推荐
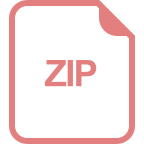
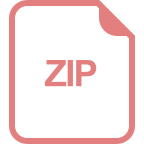
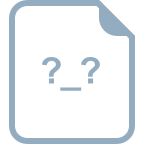
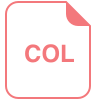
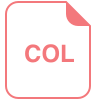
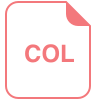
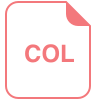
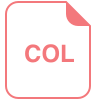









