再重新写一份俄罗斯方块游戏代码;要求:当方块下到最底端时便不能继续向下移动
时间: 2023-12-20 16:03:41 浏览: 68
好的,下面是一份简单的俄罗斯方块游戏代码,满足题目要求:
```python
import pygame
import random
# 游戏区域大小
WIDTH = 300
HEIGHT = 600
# 方块大小
BLOCK_SIZE = 30
# 游戏区域大小(格子数)
ROWS = HEIGHT // BLOCK_SIZE
COLS = WIDTH // BLOCK_SIZE
# 方块移动的时间间隔(毫秒)
MOVE_INTERVAL = 500
# 颜色定义
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
YELLOW = (255, 255, 0)
CYAN = (0, 255, 255)
MAGENTA = (255, 0, 255)
# 方块类型定义
BLOCK_TYPES = [
[(1, 1, 1, 1)], # I型方块
[(1, 1, 0), (0, 1, 1)], # Z型方块
[(0, 1, 1), (1, 1, 0)], # S型方块
[(1, 1, 1), (0, 1, 0)], # T型方块
[(1, 1, 1), (1, 0, 0)], # L型方块
[(1, 1, 1), (0, 0, 1)], # J型方块
[(1, 1), (1, 1)], # O型方块
]
# 方块颜色定义
BLOCK_COLORS = [
YELLOW, # I型方块
RED, # Z型方块
GREEN, # S型方块
CYAN, # T型方块
BLUE, # L型方块
MAGENTA, # J型方块
WHITE, # O型方块
]
# 初始化游戏
pygame.init()
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption('俄罗斯方块')
# 创建字体对象
font = pygame.font.Font(None, 36)
# 生成一个新的方块
def generate_block():
global current_block, next_block, block_x, block_y, block_type, block_color
# 将下一个方块作为当前方块
current_block = next_block
# 随机生成下一个方块
block_type = random.randint(0, len(BLOCK_TYPES) - 1)
block_color = BLOCK_COLORS[block_type]
next_block = BLOCK_TYPES[block_type]
# 方块初始位置在游戏区域顶部中央
block_x = COLS // 2 - len(current_block[0]) // 2
block_y = 0
# 绘制游戏区域
def draw_board():
for row in range(ROWS):
for col in range(COLS):
if board[row][col] != None:
# 绘制已有方块
pygame.draw.rect(screen, board[row][col], (col * BLOCK_SIZE, row * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
else:
# 绘制网格线
pygame.draw.rect(screen, WHITE, (col * BLOCK_SIZE, row * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE), 1)
# 绘制方块
def draw_block():
for row in range(len(current_block)):
for col in range(len(current_block[0])):
if current_block[row][col] == 1:
pygame.draw.rect(screen, block_color, ((col + block_x) * BLOCK_SIZE, (row + block_y) * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 方块向下移动
def move_down():
global block_x, block_y, current_block, is_bottom
# 判断方块是否已经到达底部或者下方已经有方块存在
if block_y + len(current_block) == ROWS or board[block_y + len(current_block)][block_x:block_x + len(current_block[0])] != [None] * len(current_block[0]):
# 停止方块继续下移
board[block_y:block_y + len(current_block)][block_x:block_x + len(current_block[0])] = [[block_color] * len(current_block[0])] * len(current_block)
current_block = None
# 检查是否有满行方块
eliminate_full_rows()
# 生成新方块
generate_block()
is_bottom = True
else:
# 继续下移方块
block_y += 1
# 消除满行方块
def eliminate_full_rows():
global score
# 找到满行方块
full_rows = []
for row in range(ROWS):
if None not in board[row]:
full_rows.append(row)
# 消除满行方块
for row in full_rows:
board.pop(row)
board.insert(0, [None] * COLS)
# 更新得分
score += len(full_rows) ** 2
# 显示得分
def show_score():
score_text = font.render('SCORE: ' + str(score), True, WHITE)
screen.blit(score_text, (10, 10))
# 游戏主循环
clock = pygame.time.Clock()
board = [[None] * COLS for row in range(ROWS)]
current_block = None
next_block = None
block_x = 0
block_y = 0
block_type = 0
block_color = None
is_bottom = False
score = 0
generate_block()
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if current_block is not None:
if event.key == pygame.K_LEFT:
# 左移方块
if block_x > 0 and board[block_y:block_y + len(current_block)][block_x - 1:block_x + len(current_block[0]) - 1] == [[None] * len(current_block[0])] * len(current_block):
block_x -= 1
elif event.key == pygame.K_RIGHT:
# 右移方块
if block_x + len(current_block[0]) < COLS and board[block_y:block_y + len(current_block)][block_x + 1:block_x + len(current_block[0]) + 1] == [[None] * len(current_block[0])] * len(current_block):
block_x += 1
elif event.key == pygame.K_UP:
# 旋转方块
new_block = [[current_block[col][row] for col in range(len(current_block))] for row in range(len(current_block[0]) - 1, -1, -1)]
if block_x + len(new_block[0]) <= COLS and board[block_y:block_y + len(new_block)][block_x:block_x + len(new_block[0])] == [[None] * len(new_block[0])] * len(new_block):
current_block = new_block
elif event.key == pygame.K_DOWN:
# 加速方块下落
MOVE_INTERVAL = 100
# 绘制游戏界面
screen.fill(BLACK)
draw_board()
if current_block is not None:
draw_block()
# 方块自动向下移动
if pygame.time.get_ticks() % MOVE_INTERVAL == 0:
move_down()
show_score()
pygame.display.update()
clock.tick(60)
```
这份代码使用了 Pygame 库来实现游戏界面的绘制以及事件处理,游戏主循环中的代码会不断检测当前方块是否已经到达底部或者下方已经有方块存在,如果是,则停止方块继续下移。
阅读全文
相关推荐
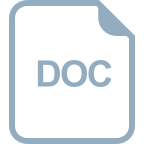
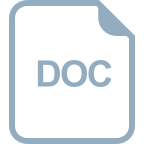
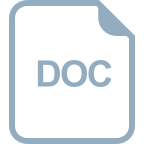
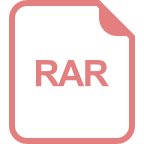
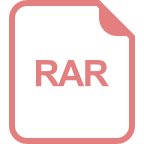
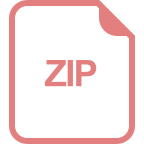
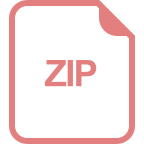
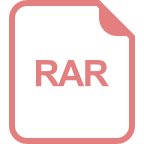
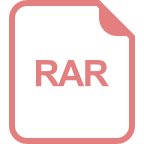
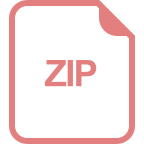
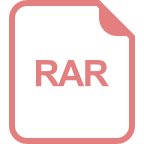
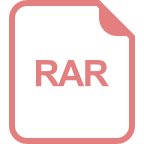
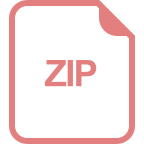
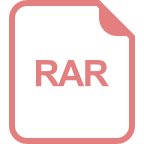
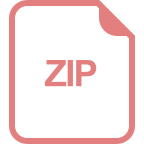
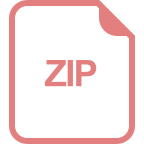
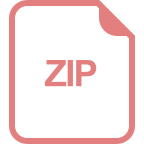