米诺陶迷宫寻找最短路径,避开怪物c++代码
时间: 2024-10-13 10:00:59 浏览: 57
米诺陶迷宫问题通常是一个经典的搜索算法问题,可以使用深度优先搜索(Depth First Search, DFS) 或者广度优先搜索(Breadth First Search, BFS) 来解决。其中BFS更适用于找寻最短路径。下面是一个简单的C++代码示例,使用邻接矩阵表示迷宫,避免怪物即遇到障碍物的情况:
```cpp
#include <iostream>
#include <vector>
#include <queue>
// 定义迷宫矩阵中的元素类型
enum CellState { EMPTY, OBSTACLE, MONSTER };
class MinoTaurosLabyrinth {
private:
std::vector<std::vector<CellState>> maze;
int width, height;
public:
// 构造函数
MinoTaurosLabyrinth(int _width, int _height, const std::vector<std::vector<int>>& grid)
: width(_width), height(_height), maze(_width, std::vector<CellState>(_height))
{
for (int i = 0; i < _height; ++i) {
for (int j = 0; j < _width; ++j) {
maze[j][i] = grid[i][j] == 0 ? EMPTY : OBSTACLE;
}
}
}
// 检查某个位置是否为空地并且不是怪物
bool isValidPosition(int x, int y) const {
return x >= 0 && x < width && y >= 0 && y < height && maze[x][y] == EMPTY;
}
// 搜索最短路径并返回结果
std::pair<int, int> findShortestPath(int start_x, int start_y) {
std::vector<std::pair<int, int>> exploredPaths;
std::queue<std::pair<int, int>> q;
q.push({start_x, start_y});
while (!q.empty()) {
auto [x, y] = q.front();
q.pop();
if (maze[x][y] != OBSTACLE) {
// 如果到达目的地,返回坐标
if (x == width - 1 && y == height - 1) {
return {x, y};
}
exploredPaths.push_back({x, y});
// 添加周围未探索的位置到队列
for (auto [dx, dy] : {{-1, 0}, {1, 0}, {0, -1}, {0, 1}}) {
if (isValidPosition(x + dx, y + dy)) {
q.push({x + dx, y + dy});
}
}
}
}
// 没有找到路径,返回空
return {-1, -1};
}
};
int main() {
int mazeWidth, mazeHeight;
std::cin >> mazeWidth >> mazeHeight;
std::vector<std::vector<int>> grid(mazeHeight, std::vector<int>(mazeWidth));
// 从输入读取迷宫矩阵
MinoTaurosLabyrinth labyrinth(mazeWidth, mazeHeight, grid);
int startX, startY;
std::cin >> startX >> startY;
auto result = labyrinth.findShortestPath(startX, startY);
if (result.first != -1) {
std::cout << "Shortest path found at (" << result.first << ", " << result.second << ")\n";
} else {
std::cout << "No path to escape from the monster!\n";
}
return 0;
}
```
在这个代码中,我们首先创建了一个MinoTaurosLabyrinth类,用于存储迷宫信息,并实现了搜索功能。然后在main函数中,从用户输入读取迷宫信息和起点,最后找出最短路径。
阅读全文
相关推荐


















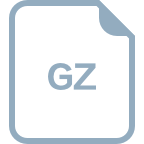