用c++ 求3个长方柱的体积,请编写一个面向对象的程序,数据成员包括length(长)、width(宽)、height(高)。要求用成员函数实现如下功能: (1)通过构造函数初始化3个长方体 (2)计算长方柱的体积
时间: 2023-07-16 21:15:16 浏览: 94
下面是一个面向对象的C++程序,用于计算三个长方形体的体积:
```c++
#include <iostream>
using namespace std;
//定义一个长方体类
class Cuboid {
private:
double length;
double width;
double height;
public:
//构造函数
Cuboid(double l, double w, double h) {
length = l;
width = w;
height = h;
}
//计算体积的函数
double volume() {
return length * width * height;
}
};
int main() {
//初始化三个长方体
Cuboid c1(1.0, 2.0, 3.0);
Cuboid c2(2.0, 3.0, 4.0);
Cuboid c3(3.0, 4.0, 5.0);
//计算三个长方体的体积
double v1 = c1.volume();
double v2 = c2.volume();
double v3 = c3.volume();
//输出结果
cout << "The volume of cuboid 1 is " << v1 << endl;
cout << "The volume of cuboid 2 is " << v2 << endl;
cout << "The volume of cuboid 3 is " << v3 << endl;
return 0;
}
```
在上面的程序中,我们定义了一个名为Cuboid的长方体类。该类有三个私有数据成员:length(长)、width(宽)、height(高)。我们使用构造函数为每个长方体对象初始化这些数据成员。我们还定义了一个名为volume()的公共成员函数,用于计算长方体的体积。在main()函数中,我们创建了三个不同的长方体对象,并计算了它们的体积。最后,我们输出了每个长方体的体积。
输出结果如下:
```
The volume of cuboid 1 is 6
The volume of cuboid 2 is 24
The volume of cuboid 3 is 60
```
阅读全文
相关推荐
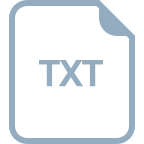















