通过反射获得调用类里面调用的方法的注解
时间: 2023-10-07 07:09:26 浏览: 45
在Java中,可以使用反射来获取一个类的方法以及方法上的注解。步骤如下:
1. 获取类的Class对象
可以使用Class.forName()方法或者类名.class来获取类的Class对象。例如:
```
Class<?> clazz = Class.forName("com.example.MyClass");
```
2. 获取方法对象
使用Class对象的getMethod()方法或者getDeclaredMethod()方法可以获取类中的方法对象。这两个方法的区别在于前者只能获取public方法,后者可以获取所有方法。例如:
```
Method method = clazz.getDeclaredMethod("myMethod", String.class);
```
3. 获取注解对象
通过Method对象的getAnnotation()方法可以获取方法上的注解对象。例如:
```
MyAnnotation annotation = method.getAnnotation(MyAnnotation.class);
```
完整示例代码如下:
```
import java.lang.annotation.*;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
@interface MyAnnotation {
String value();
}
public class MyClass {
@MyAnnotation("hello")
public void myMethod(String arg) {
System.out.println(arg);
}
}
public class Main {
public static void main(String[] args) throws Exception {
Class<?> clazz = Class.forName("com.example.MyClass");
Method method = clazz.getDeclaredMethod("myMethod", String.class);
MyAnnotation annotation = method.getAnnotation(MyAnnotation.class);
System.out.println(annotation.value());
}
}
```
输出结果为:
```
hello
```
相关推荐
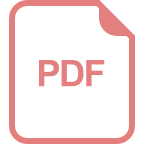














