利用顺序查找,二分查找,二叉排序树查找实现对整数的查找,c语言完整程序
时间: 2023-07-18 13:34:47 浏览: 98
好的,这里是一个完整的程序,其中包括了顺序查找、二分查找、二叉排序树查找的实现:
```c
#include <stdio.h>
#include <stdlib.h>
/* 顺序查找 */
int sequentialSearch(int arr[], int n, int x) {
for(int i=0; i<n; i++) {
if(arr[i] == x) {
return i;
}
}
return -1; //未找到
}
/* 二分查找 */
int binarySearch(int arr[], int l, int r, int x) {
if(r >= l) {
int mid = l + (r-l)/2;
if(arr[mid] == x) {
return mid;
}
if(arr[mid] > x) {
return binarySearch(arr, l, mid-1, x);
}
return binarySearch(arr, mid+1, r, x);
}
return -1; //未找到
}
/* 二叉排序树 */
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
TreeNode* insert(TreeNode* root, int val) {
if(root == NULL) {
TreeNode* node = (TreeNode*)malloc(sizeof(TreeNode));
node->val = val;
node->left = NULL;
node->right = NULL;
return node;
}
if(val < root->val) {
root->left = insert(root->left, val);
} else if(val > root->val) {
root->right = insert(root->right, val);
}
return root;
}
TreeNode* search(TreeNode* root, int val) {
if(root == NULL || root->val == val) {
return root;
}
if(val < root->val) {
return search(root->left, val);
}
return search(root->right, val);
}
void inorderTraversal(TreeNode* root) {
if(root != NULL) {
inorderTraversal(root->left);
printf("%d ", root->val);
inorderTraversal(root->right);
}
}
int main() {
int arr[] = {2, 5, 1, 8, 3, 9, 4, 6, 7};
int n = sizeof(arr)/sizeof(arr[0]);
int x = 5;
/* 顺序查找 */
int index = sequentialSearch(arr, n, x);
if(index != -1) {
printf("%d found at index %d by sequential search.\n", x, index);
} else {
printf("%d not found by sequential search.\n", x);
}
/* 二分查找 */
index = binarySearch(arr, 0, n-1, x);
if(index != -1) {
printf("%d found at index %d by binary search.\n", x, index);
} else {
printf("%d not found by binary search.\n", x);
}
/* 二叉排序树 */
TreeNode* root = NULL;
for(int i=0; i<n; i++) {
root = insert(root, arr[i]);
}
inorderTraversal(root);
TreeNode* node = search(root, x);
if(node != NULL) {
printf("\n%d found in the tree by binary search tree!", node->val);
} else {
printf("\n%d not found in the tree by binary search tree!", x);
}
return 0;
}
```
希望可以帮到您!
阅读全文
相关推荐
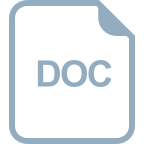
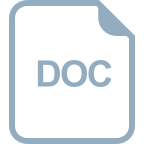
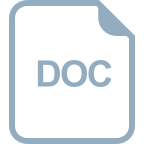

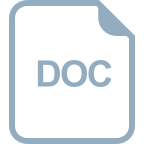
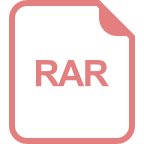
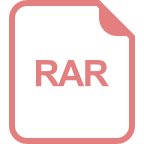
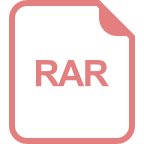
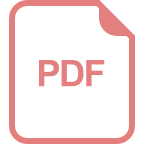
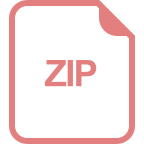
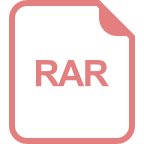
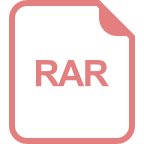
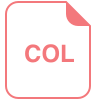
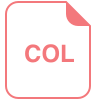
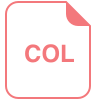
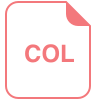
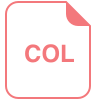
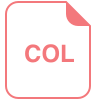
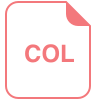
