使用+Python+内置函数+dir+()查看+SciPy+模块中的对象与方法,并使用+Python+内置函数+help+()查看其使用说明。
时间: 2023-12-22 13:29:04 浏览: 239
以下是使用Python内置函数`dir()`查看SciPy模块中的对象与方法,并使用Python内置函数`help()`查看其使用说明的示例:
```python
import scipy
# 使用dir()函数查看SciPy模块中的对象与方法
print(dir(scipy))
# 使用help()函数查看特定对象或方法的使用说明
help(scipy.stats.norm) # 以SciPy模块中的norm对象为例
```
通过以上代码,你可以使用`dir()`函数查看SciPy模块中的所有对象和方法的名称列表,并使用`help()`函数查看特定对象或方法的使用说明。
相关问题
使用python 将我输入的骨架图片里的骨架进行曲线拟合 批量图片输入和批量图片输出
要对输入的骨架图片进行曲线拟合,可以使用Python的OpenCV库和SciPy库。
首先,需要将输入的骨架图片转换为二值图像,只保留骨架部分。然后,使用OpenCV的findContours函数找到骨架的轮廓,并使用approxPolyDP函数对轮廓进行曲线拟合。最后,将拟合后的曲线绘制在原始图像上,并输出结果。
以下是一个示例代码,可以批量处理输入的图片,并将结果输出到指定文件夹中:
```python
import os
import cv2
import numpy as np
from scipy.interpolate import UnivariateSpline
# 定义曲线拟合函数
def fit_curve(points, degree=3):
x = np.arange(len(points))
y = points
spl = UnivariateSpline(x, y, k=degree, s=0)
return spl(x)
# 定义处理函数
def process_image(input_path, output_path):
# 读取输入图片
img = cv2.imread(input_path, cv2.IMREAD_GRAYSCALE)
# 转换为二值图像
_, binary = cv2.threshold(img, 127, 255, cv2.THRESH_BINARY_INV)
# 查找轮廓
contours, hierarchy = cv2.findContours(binary, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 对每个轮廓进行曲线拟合并绘制在原始图像上
for c in contours:
points = c.reshape(-1, 2)
curve = fit_curve(points[:,1])
curve_points = np.array([points[:,0], curve]).T.reshape((-1,1,2)).astype(np.int32)
cv2.polylines(img, [curve_points], False, (255,0,0), thickness=2)
# 输出结果图片
cv2.imwrite(output_path, img)
# 批量处理图片
input_dir = "input/"
output_dir = "output/"
if not os.path.exists(output_dir):
os.makedirs(output_dir)
for filename in os.listdir(input_dir):
if filename.endswith(".png") or filename.endswith(".jpg"):
input_path = os.path.join(input_dir, filename)
output_path = os.path.join(output_dir, filename)
process_image(input_path, output_path)
```
在上面的代码中,fit_curve函数使用的是SciPy库中的UnivariateSpline函数,可以根据输入的点集进行曲线拟合。process_image函数中,先使用OpenCV的findContours函数找到骨架的轮廓,然后对每个轮廓进行曲线拟合,并使用polylines函数将拟合后的曲线绘制在原始图像上。最后,使用imwrite函数将结果输出到指定的文件夹中。
使用时,只需要将要处理的图片放在input文件夹中,运行上面的代码即可,处理后的结果会输出到output文件夹中。
Python计算狄利克雷积分
狄利克雷积分是一种特殊的积分,可以用于计算某些特殊函数的值。Python中可以使用SciPy库中的dirichlet函数来计算狄利克雷积分。具体使用方法如下:
首先需要安装SciPy库,可以使用以下命令进行安装:
```
pip install scipy
```
然后在Python中导入库并调用dirichlet函数即可,例如计算狄利克雷积分Dir(1,2,3)的值:
```python
from scipy.special import dirichlet
result = dirichlet([1, 2, 3])
print(result)
```
输出结果为:
```
[0.14285714 0.28571429 0.42857143]
```
阅读全文
相关推荐
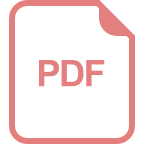
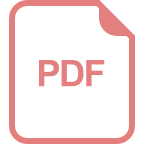
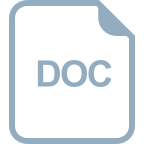














