C# 生成随机正弦曲线
时间: 2024-11-04 20:09:19 浏览: 15
在C#中生成随机正弦曲线,可以使用System.Drawing命名空间中的Graphics类来绘制图形。以下是一个简单的示例代码,展示如何在Windows窗体应用程序中生成一个随机的正弦曲线:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
public class SineWaveForm : Form
{
private Timer timer;
private float phase = 0;
private Random random = new Random();
public SineWaveForm()
{
this.Width = 800;
this.Height = 600;
this.Text = "随机正弦曲线";
timer = new Timer();
timer.Interval = 100; // 更新频率为100毫秒
timer.Tick += OnTimerTick;
timer.Start();
}
private void OnTimerTick(object sender, EventArgs e)
{
phase += (float)(random.NextDouble() * 2 - 1); // 随机改变相位
this.Invalidate(); // 请求重绘窗体
}
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
DrawSineWave(e.Graphics);
}
private void DrawSineWave(Graphics g)
{
int width = this.ClientSize.Width;
int height = this.ClientSize.Height;
int amplitude = height / 4; // 振幅
int frequency = 5; // 频率
PointF[] points = new PointF[width];
for (int x = 0; x < width; x++)
{
float y = (float)(amplitude * Math.Sin((x / (float)frequency) + phase));
points[x] = new PointF(x, height / 2 + y);
}
g.Clear(Color.White);
g.DrawLines(Pens.Black, points);
}
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new SineWaveForm());
}
}
```
这段代码创建了一个名为`SineWaveForm`的窗体类,其中包含一个定时器用于周期性地更新正弦波的相位,从而产生动态效果。`DrawSineWave`方法负责根据当前相位计算正弦波的点并绘制到窗体上。每次定时器触发时,都会调用`Invalidate`方法请求重绘窗体,从而更新显示的正弦波。
阅读全文
相关推荐
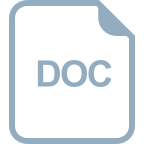
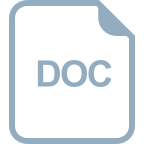
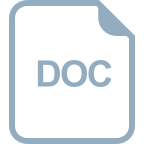
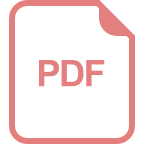
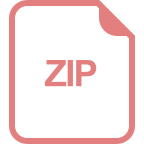
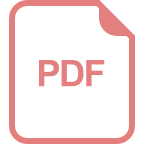
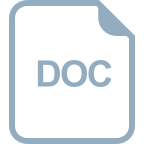
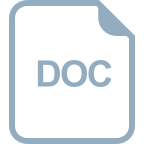
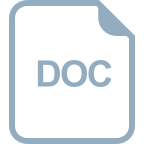
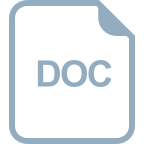
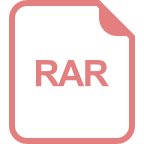
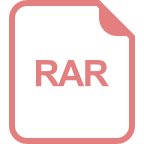
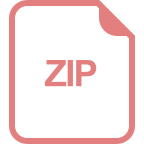
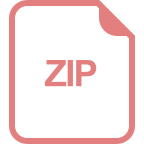
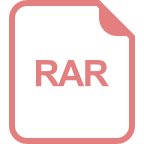