tab = Tab() tab.add(showBar(), "笔记本电脑总销量排行榜") tab.add(showPie1(), "MacBook 京东自营店铺销量分析") tab.add(showPie2(), "各品牌4999到10099笔记本销量占比") tab.add(showPie3(), "笔记本电脑全价位各品牌销量占比") tab.add(showBar2(),"好评率从高到低笔记本电脑销量") tab.add(showLine(), "销量Top 10品牌笔记本电脑均价") tab.add(showWordCould(), "品牌词云图") tab.add(showPoint(), "京东各店铺在售品牌") tab.render("JD_phone.html")
时间: 2023-09-24 21:09:02 浏览: 90
这段代码使用了 Python 中的 Tab 和各种图表函数来生成一个包含多个图表的 HTML 页面。具体来说,它创建了一个 Tab 对象,然后使用 add 方法将各个图表添加到该对象中,并为每个图表指定一个标签名。最后,调用 render 方法将 Tab 对象转换为 HTML 页面并保存到本地文件 "JD_phone.html" 中。
相关问题
import numpy as np import matplotlib.pyplot as plt plt.rcParams['font.sans-serif'] = ['SimHei'] plt.rcParams['axes.unicode_minus'] = False men_means = (85.5, 91, 72, 59, 66, 55) women_means = (94, 82, 89.5, 62, 49, 53) ind = np.arange(len(men_means)) width = 0.2 fig = plt.figure() ax = fig.add_subplot(111) ax.bar(ind - width / 2, men_means, width, label='男生平均成绩') ax.bar(ind + 0.2, women_means, width, label='女生平均成绩') ax.set_title('高二男生女生各科平均成绩') ax.set_xticks(ind) ax.set_xticklabels(['语文', '数学', '英语', '物理', '化学', '生物']) plt.ylim(40, 100) ax.legend() plt.show()美化并转为环形图
以下是美化并转为环形图的代码:
```
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.patches import ConnectionPatch
plt.rcParams['font.sans-serif'] = ['SimHei']
plt.rcParams['axes.unicode_minus'] = False
men_means = (85.5, 91, 72, 59, 66, 55)
women_means = (94, 82, 89.5, 62, 49, 53)
ind = np.arange(len(men_means))
width = 0.2
fig, ax = plt.subplots(figsize=(8, 8))
ax.bar(ind - width / 2, men_means, width, label='男生平均成绩', color='tab:blue')
ax.bar(ind + 0.2, women_means, width, label='女生平均成绩', color='tab:orange')
ax.set_title('高二男生女生各科平均成绩', fontsize=20)
ax.set_xticks(ind)
ax.set_xticklabels(['语文', '数学', '英语', '物理', '化学', '生物'], fontsize=15)
ax.set_ylim(40, 100)
ax.legend(fontsize=15)
# 环形图部分
theta = np.linspace(0, 2 * np.pi, len(men_means) + 1)
radii = np.repeat(1.5, len(men_means) + 1)
ax1 = plt.subplot(111, projection='polar')
ax1.plot(theta, radii, color='black', linewidth=1)
for i in range(len(men_means)):
ax1.fill_between([theta[i], theta[i+1]], 0, radii[i+1], color='tab:blue', alpha=0.6)
ax1.fill_between([theta[i], theta[i+1]], 0, radii[i], color='tab:orange', alpha=0.6)
posA = ax1.transData.transform((theta[i], radii[i]))
posB = ax1.transData.transform((theta[i], radii[i+1]))
coordsA = ax.transData.inverted().transform(posA)
coordsB = ax.transData.inverted().transform(posB)
con = ConnectionPatch(xyA=coordsA, xyB=coordsB, coordsA="data", coordsB="data", lw=1, color='black', alpha=0.8)
fig.add_artist(con)
plt.tight_layout()
plt.show()
```
这个代码首先绘制了原始的柱状图,然后使用极坐标系绘制了环形图。环形图中,每个扇形的角度与柱状图中每个柱子对应,颜色则代表男女生的成绩。同时,为了使环形图与柱状图对应,我们使用 ConnectionPatch 将两个部分连接起来。最后,使用 tight_layout() 函数调整布局,使得图像更加美观。
用java写一个底部导航栏+Tab选项卡的代码
以下是使用Java代码实现底部导航栏和Tab选项卡的示例:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class BottomNavigationBar extends JFrame implements ActionListener {
private JPanel bottomPanel, tabPanel;
private JButton homeButton, searchButton, profileButton;
private JLabel homeLabel, searchLabel, profileLabel;
private CardLayout cardLayout;
public BottomNavigationBar() {
initComponents();
}
private void initComponents() {
setTitle("Bottom Navigation Bar");
bottomPanel = new JPanel(new BorderLayout());
tabPanel = new JPanel(new GridLayout(1, 3));
homeButton = new JButton(new ImageIcon("home.png"));
searchButton = new JButton(new ImageIcon("search.png"));
profileButton = new JButton(new ImageIcon("profile.png"));
homeLabel = new JLabel("Home");
searchLabel = new JLabel("Search");
profileLabel = new JLabel("Profile");
homeButton.addActionListener(this);
searchButton.addActionListener(this);
profileButton.addActionListener(this);
tabPanel.add(homeButton);
tabPanel.add(searchButton);
tabPanel.add(profileButton);
bottomPanel.add(tabPanel, BorderLayout.CENTER);
bottomPanel.add(homeLabel, BorderLayout.WEST);
bottomPanel.add(searchLabel, BorderLayout.CENTER);
bottomPanel.add(profileLabel, BorderLayout.EAST);
cardLayout = new CardLayout();
JPanel contentPanel = new JPanel(cardLayout);
contentPanel.add(new JLabel("Home Screen"), "Home");
contentPanel.add(new JLabel("Search Screen"), "Search");
contentPanel.add(new JLabel("Profile Screen"), "Profile");
add(bottomPanel, BorderLayout.SOUTH);
add(contentPanel, BorderLayout.CENTER);
setSize(500, 500);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == homeButton) {
cardLayout.show(tabPanel, "Home");
} else if (e.getSource() == searchButton) {
cardLayout.show(tabPanel, "Search");
} else if (e.getSource() == profileButton) {
cardLayout.show(tabPanel, "Profile");
}
}
public static void main(String[] args) {
new BottomNavigationBar();
}
}
```
在这个示例中,底部导航栏由三个按钮和标签组成,分别用于在主屏幕,搜索屏幕和个人资料屏幕之间进行切换。CardLayout用于管理不同屏幕之间的切换。当用户点击底部导航栏上的一个按钮时,相应的屏幕将显示在中心面板上。
阅读全文
相关推荐
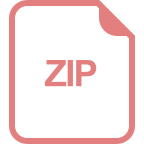
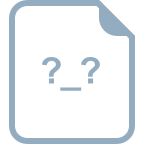
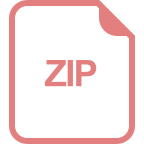
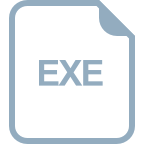
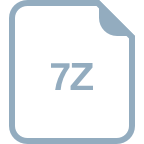
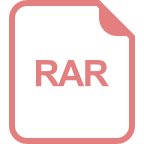
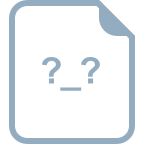
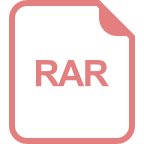
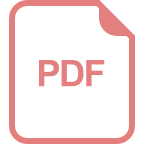
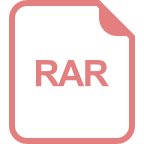
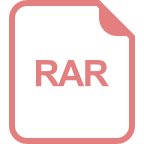
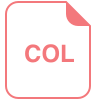
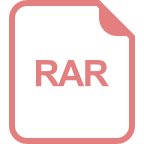