python代码更新高程点shp文件,提取出每个点对应的SRTM.tif和area.tif内的高程值,存入srtm和dsm两个字段中。
时间: 2024-05-13 21:17:40 浏览: 281
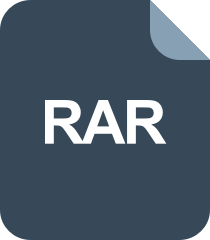
获取经纬度点的高程值
这里提供一个Python脚本,可以实现更新高程点shp文件的功能,并提取出每个点对应的SRTM.tif和area.tif内的高程值,存入srtm和dsm两个字段中。需要使用到GDAL和Fiona这两个库。
```python
import os
import fiona
import gdal
# 设置输入输出路径
input_dir = '/path/to/input/dir'
output_dir = '/path/to/output/dir'
# 打开高程点shp文件
with fiona.open(os.path.join(input_dir, 'elevation_points.shp'), 'r') as points:
# 获取SRTM.tif和area.tif的路径
srtm_path = os.path.join(input_dir, 'SRTM.tif')
dsm_path = os.path.join(input_dir, 'area.tif')
# 打开SRTM.tif和area.tif
srtm_ds = gdal.Open(srtm_path)
dsm_ds = gdal.Open(dsm_path)
# 获取SRTM.tif和area.tif的波段
srtm_band = srtm_ds.GetRasterBand(1)
dsm_band = dsm_ds.GetRasterBand(1)
# 获取高程点shp文件的元数据
meta = points.meta
# 将srtm和dsm两个字段添加到元数据中
meta['schema']['properties']['srtm'] = 'float'
meta['schema']['properties']['dsm'] = 'float'
# 创建新的高程点shp文件
with fiona.open(os.path.join(output_dir, 'elevation_points_updated.shp'), 'w', **meta) as output:
# 遍历每个高程点
for point in points:
# 获取高程点的坐标
x, y = point['geometry']['coordinates']
# 根据坐标获取SRTM.tif和area.tif中的像素值
srtm_value = srtm_band.ReadAsArray(int(x), int(y), 1, 1)[0][0]
dsm_value = dsm_band.ReadAsArray(int(x), int(y), 1, 1)[0][0]
# 将像素值写入高程点的srtm和dsm字段中
point['properties']['srtm'] = srtm_value
point['properties']['dsm'] = dsm_value
# 将更新后的高程点写入新的shp文件中
output.write(point)
```
使用时需要将代码中的`/path/to/input/dir`和`/path/to/output/dir`替换为实际的输入和输出路径。如果需要更新其他字段,可以根据需要修改代码。
阅读全文
相关推荐
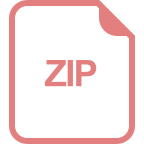
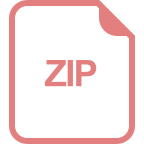
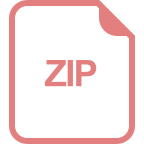
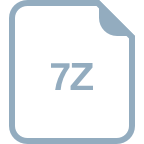
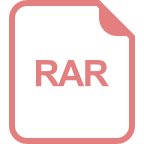
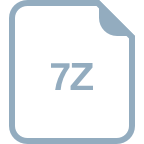
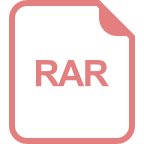
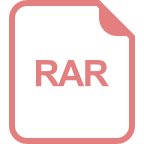
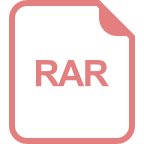
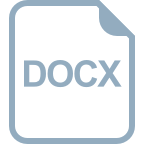
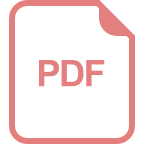
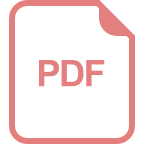
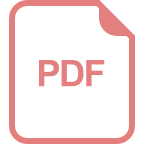
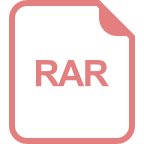
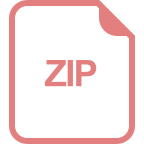
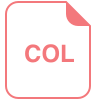