怎样通过Java语言编写链式表
时间: 2024-09-13 11:09:29 浏览: 35
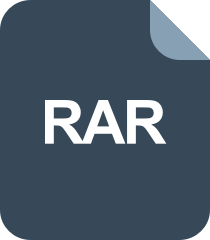
Java模板语言 Beetl
在Java中,我们可以使用自定义类来创建链表,并通过链表节点的设计来实现链式的操作。链表通常包含三个关键部分:节点(Node)、头结点(Head)和链接(Link)。下面是一个简单的单向链表的实现示例:
```java
// 链接节点定义
class Node {
int data; // 节点存储的数据
Node next; // 指向下一个节点的引用
public Node(int data) {
this.data = data;
this.next = null;
}
}
// 链表类
public class LinkedList {
private Node head;
// 添加节点到链表头部
public void addFirst(int data) {
Node newNode = new Node(data);
newNode.next = head;
head = newNode;
}
// 添加节点到链表尾部
public void addLast(int data) {
if (head == null) {
head = new Node(data);
return;
}
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = new Node(data);
}
// 删除特定值的节点
public void remove(int value) {
if (head == null) return;
if (head.data == value) {
head = head.next;
return;
}
Node current = head;
while (current.next != null && current.next.data != value) {
current = current.next;
}
if (current.next != null) {
current.next = current.next.next;
}
}
// 打印链表
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
Node node = head;
while (node != null) {
sb.append(node.data + " -> ");
node = node.next;
}
return sb.substring(0, sb.length() - 4); // 去掉最后一个" -> "
}
}
```
阅读全文
相关推荐
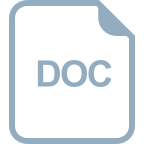
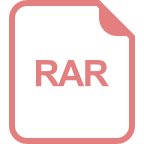


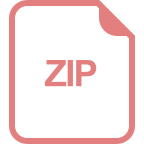
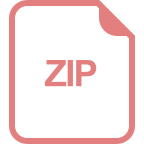
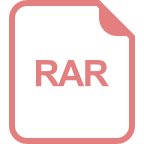
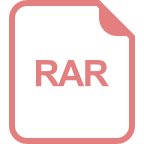
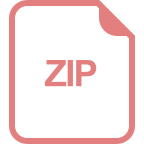
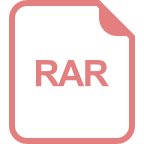
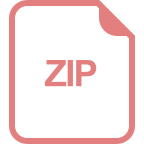
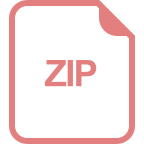
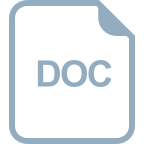
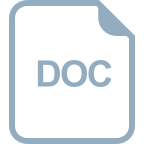
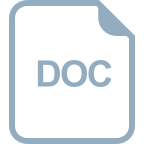
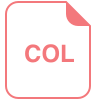