c语言比较两个ipv6地址的大小
时间: 2024-05-12 13:19:18 浏览: 27
比较两个IPv6地址的大小需要将其转换为数值进行比较。以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <string.h>
#include <arpa/inet.h>
int ipv6cmp(struct in6_addr *a, struct in6_addr *b) {
for (int i = 0; i < 16; i++) {
if (a->s6_addr[i] < b->s6_addr[i]) {
return -1;
} else if (a->s6_addr[i] > b->s6_addr[i]) {
return 1;
}
}
return 0;
}
int main() {
struct in6_addr a, b;
memset(&a, 0, sizeof(struct in6_addr));
memset(&b, 0, sizeof(struct in6_addr));
inet_pton(AF_INET6, "2001:db8::1", &a);
inet_pton(AF_INET6, "2001:db8::2", &b);
int cmp = ipv6cmp(&a, &b);
if (cmp < 0) {
printf("a < b\n");
} else if (cmp > 0) {
printf("a > b\n");
} else {
printf("a = b\n");
}
return 0;
}
```
在上面的示例中,我们定义了一个`ipv6cmp`函数,它接受两个`struct in6_addr`类型的参数,并将它们转换为数值进行比较。如果第一个参数小于第二个参数,则返回-1;如果第一个参数大于第二个参数,则返回1;否则返回0。
在`main`函数中,我们使用`inet_pton`函数将IPv6地址转换为`struct in6_addr`类型的变量,并将它们传递给`ipv6cmp`函数进行比较。最后根据比较结果输出相应的信息。
相关推荐
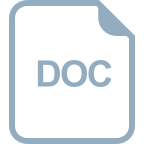
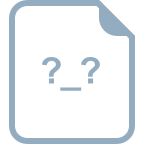
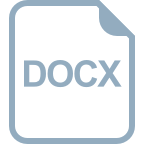














