协程风格socket demo 包括收发消息
时间: 2023-08-02 11:12:11 浏览: 42
以下是一个使用协程风格实现的简单的 Socket Demo,其中包括收发消息的功能。这个 Demo 使用 Python 的 asyncio 模块实现协程。
```python
import asyncio
async def handle_client(reader, writer):
while True:
data = await reader.read(1024)
if not data:
break
message = data.decode().strip()
print(f"Received message: {message}")
response = f"You said: {message}"
writer.write(response.encode())
await writer.drain()
writer.close()
async def main():
server = await asyncio.start_server(handle_client, '127.0.0.1', 8888)
async with server:
await server.serve_forever()
asyncio.run(main())
```
在这个 Demo 中,我们使用 asyncio.start_server() 函数创建了一个服务器,监听本地的 8888 端口。当有客户端连接时,handle_client() 协程会被调用来处理连接。
在 handle_client() 函数中,我们首先读取客户端发送的数据,然后将其打印出来。接着,我们构建了一个简单的响应消息,并将其发送回客户端。最后,我们关闭了连接。
在 main() 函数中,我们使用 asyncio.run() 来运行整个程序。这个函数会自动创建一个新的事件循环,并运行其中的协程。
现在,我们可以使用 telnet 或其他工具来连接到这个服务器,并发送消息。例如:
```
$ telnet 127.0.0.1 8888
Trying 127.0.0.1...
Connected to localhost.
Escape character is '^]'.
Hello, server!
You said: Hello, server!
```
上面的例子只是一个简单的演示。在实际应用中,我们可能需要更复杂的逻辑来处理数据、连接管理等等。但是,协程风格的代码可以让这些逻辑更加清晰和易于维护。
相关推荐
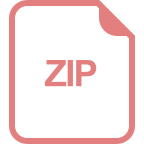














