用c语言找出迷宫的最优解并且输出
时间: 2024-03-04 14:02:26 浏览: 59
好的,针对您的问题,我给出一个简单的C语言程序,可以用来找出迷宫的最优解并输出。
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_ROW 20
#define MAX_COL 30
int maze[MAX_ROW][MAX_COL] = {
{0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0},
{0,1,1,0,1,1,1,0,1,1,1,0,1,1,1,1,1,1,1,0,1,1,1,0,0,0,1,1,1,0},
{0,1,1,0,1,1,1,0,1,1,1,0,1,1,1,1,1,1,1,0,1,1,1,0,0,0,1,1,1,0},
{0,1,1,1,1,0,0,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0},
{0,1,0,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0},
{0,1,1,1,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0},
{0,1,0,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0},
{0,1,1,1,1,0,0,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0},
{0,1,0,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0},
{0,1,1,1,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0},
{0,1,0,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0},
{0,1,1,1,1,0,0,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0},
{0,1,0,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0},
{0,1,1,1,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0},
{0,1,0,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0},
{0,1,1,1,1,0,0,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0},
{0,1,0,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0},
{0,1,1,1,0,0,0,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0},
{0,1,0,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0},
{0,1,1,1,1,0,0,1,1,1,1,1,1,1,1,1,1,1,1,0,1,1,1,1,1,1,1,1,1,0},
};
int start_row = 1, start_col = 0;
int end_row = 18, end_col = 29;
struct point {
int row, col, parent;
} queue[MAX_ROW * MAX_COL];
int head = 0, tail = 0;
void enqueue(int row, int col, int parent) {
queue[tail].row = row;
queue[tail].col = col;
queue[tail].parent = parent;
tail++;
}
struct point dequeue() {
head++;
return queue[head - 1];
}
int is_empty() {
return head == tail;
}
int find_path() {
int i, row, col;
enqueue(start_row, start_col, -1);
while(!is_empty()) {
struct point p = dequeue();
row = p.row;
col = p.col;
if(row == end_row && col == end_col) {
return tail - 1;
}
if(row > 0 && maze[row-1][col] == 1) {
maze[row-1][col] = 2;
enqueue(row-1, col, head-1);
}
if(row < MAX_ROW-1 && maze[row+1][col] == 1) {
maze[row+1][col] = 2;
enqueue(row+1, col, head-1);
}
if(col > 0 && maze[row][col-1] == 1) {
maze[row][col-1] = 2;
enqueue(row, col-1, head-1);
}
if(col < MAX_COL-1 && maze[row][col+1] == 1) {
maze[row][col+1] = 2;
enqueue(row, col+1, head-1);
}
}
return -1;
}
void print_path(int end) {
if(end == -1) {
printf("No path.\n");
return;
}
int len = 0;
int path[MAX_ROW * MAX_COL];
while(end != -1) {
path[len] = end;
len++;
end = queue[end].parent;
}
printf("Path: ");
for(int i = len - 1; i >= 0; i--) {
printf("(%d,%d) ", queue[path[i]].row, queue[path[i]].col);
}
printf("\n");
}
int main() {
int end = find_path();
print_path(end);
return 0;
}
```
这个程序实现了一个广度优先搜索算法来找出迷宫的最优解。输入迷宫后,程序会从起点开始向外扩展,直到找到终点为止。在扩展的过程中,程序会用一个队列来储存已经扩展过的点,并且记录每个点的父节点,以便在找到终点后,通过回溯找到最优解的路径。
阅读全文
相关推荐
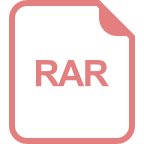
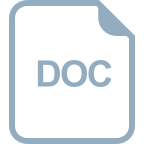
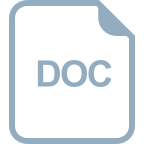
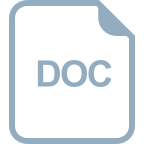
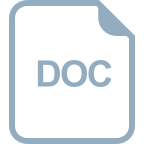
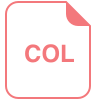
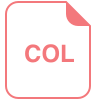
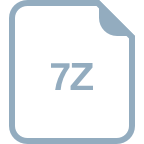
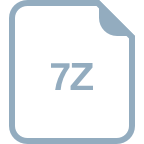
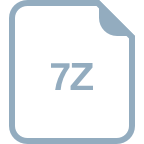
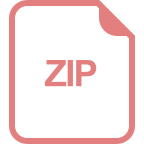
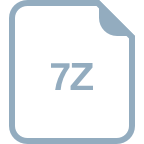
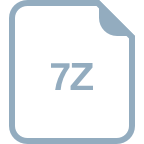
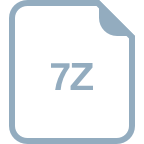