用c++构建正向的linked list,即每次在当前的linked list末尾插入新元素,构建成功后,用for循环打印该linked list,新元素要求是字符串
时间: 2024-05-01 18:22:11 浏览: 87
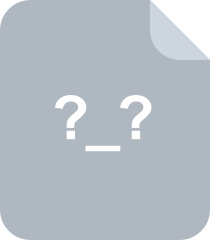
c++设计实现一个"字符串类",要求系统设计具有一定弹性和可扩展性,使得后续维护和扩展功能更容易,增加或修改系统功能变得更简单。

以下是构建正向的linked list的C++代码:
```c++
#include <iostream>
#include <string>
using namespace std;
class Node {
public:
string data;
Node* next;
Node(string data) {
this->data = data;
this->next = nullptr;
}
};
class LinkedList {
public:
Node* head;
Node* tail;
LinkedList() {
this->head = nullptr;
this->tail = nullptr;
}
void addNode(string data) {
Node* newNode = new Node(data);
if (this->head == nullptr) {
this->head = newNode;
this->tail = newNode;
}
else {
this->tail->next = newNode;
this->tail = newNode;
}
}
};
int main() {
LinkedList list;
list.addNode("Hello");
list.addNode("World");
list.addNode("!");
Node* current = list.head;
while (current != nullptr) {
cout << current->data << " ";
current = current->next;
}
return 0;
}
```
在上面的代码中,我们定义了两个类:Node和LinkedList。
Node类表示每个节点,包含一个字符串数据成员和一个指向下一个节点的指针成员。
LinkedList类表示整个链表,包含一个指向链表头部的指针成员和一个指向链表尾部的指针成员,以及一个addNode方法用于向链表末尾添加新的节点。
在主函数中,我们首先创建了一个空的LinkedList对象,并使用addNode方法向其中添加了三个节点。然后,我们使用一个while循环遍历整个链表,并依次输出每个节点的数据成员。输出结果为"Hello World !"。
阅读全文
相关推荐
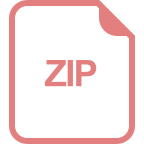


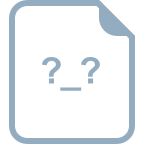
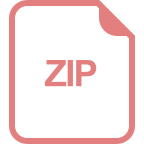
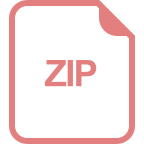
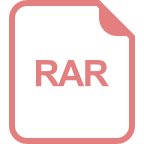
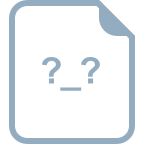
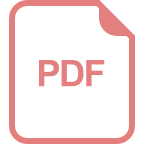
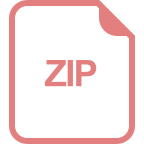




