create procedure pb_pro1(in publisher_name varchar(20),in book_name varchar(30),book_sum int,price int) -> begin -> select publishername,bookname,booksum,price -> from publisher,book -> where publisher.publisherid=book.publishid -> and publisher_name=publishername -> and book_name=bookname; -> end$$ delimiter;
时间: 2024-04-03 08:33:46 浏览: 78
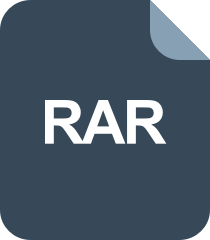
beams.rar.rar_Airy pro1_mustubl_tipyw8_艾里_艾里光
这是一个 MySQL 存储过程的代码,它的作用是根据出版商的名称和书籍的名称查询出版商和书籍的信息。存储过程的名称是 pb_pro1,它有四个输入参数,分别是 publisher_name、book_name、book_sum 和 price。其中 publisher_name 和 book_name 是用来确定查询的出版商和书籍的名称,book_sum 和 price 则是查询到的书籍的数量和价格。在查询过程中,使用了 publisher 和 book 两个表,它们之间的关联条件是 publisher.publisherid=book.publishid。最后,使用了 delimiter 关键字来指定存储过程代码的结束符。
阅读全文
相关推荐
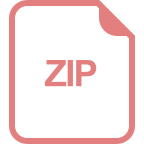
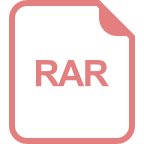
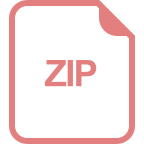
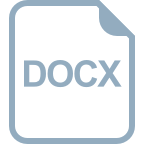
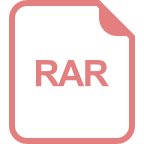
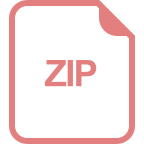
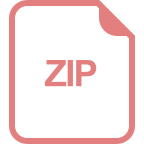
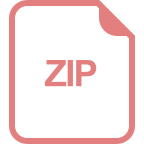
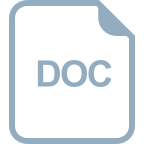
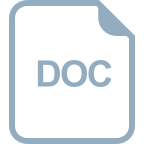






