用C语言编写代码实现多边形游戏问题。多边形每个顶点的数值a(|a|<=6)和边上的运算符可以随机生成,运行程序,记录分别对十条边,二十条边,三十条边,四十条边,所用的时间,列出表格显示结果。
时间: 2024-03-23 09:44:02 浏览: 59
好的,以下是基于C语言的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAX_VERTEX 40 // 最大的顶点数
#define MAX_TIME 1000 // 最大的运行次数
#define MAX_OPERATORS 4 // 运算符数量
#define ADDITION '+' // 加法
#define SUBTRACTION '-' // 减法
#define MULTIPLICATION '*' // 乘法
#define DIVISION '/' // 除法
typedef struct {
int vertex; // 顶点数
int time[MAX_TIME]; // 运行时间
} Record;
int calculate(int vertices[], char operators[], int count) {
int result = vertices[0];
for (int i = 0; i < count - 1; i++) {
switch (operators[i]) {
case ADDITION:
result += vertices[i + 1];
break;
case SUBTRACTION:
result -= vertices[i + 1];
break;
case MULTIPLICATION:
result *= vertices[i + 1];
break;
case DIVISION:
result /= vertices[i + 1];
break;
default:
// 非法运算符
return 0;
}
}
return result;
}
void generate_question(int vertices[], char operators[], int count) {
for (int i = 0; i < count; i++) {
vertices[i] = rand() % 13 - 6; // 生成-6到6之间的整数
operators[i] = rand() % MAX_OPERATORS;
}
}
void record_time(Record records[], int vertex, int time) {
for (int i = 0; i < MAX_TIME; i++) {
if (records[vertex - 10].time[i] == 0) {
records[vertex - 10].vertex = vertex;
records[vertex - 10].time[i] = time;
break;
}
}
}
void print_records(Record records[], int count) {
printf("Vertex\tTime (ms)\n");
for (int i = 0; i < count; i++) {
printf("%d\t", records[i].vertex);
for (int j = 0; j < MAX_TIME; j++) {
if (records[i].time[j] > 0) {
printf("%d ", records[i].time[j]);
}
}
printf("\n");
}
}
int main() {
srand(time(NULL));
Record records[MAX_VERTEX - 9] = {0}; // 初始化记录数组
for (int vertex = 10; vertex <= MAX_VERTEX; vertex += 10) {
int vertices[vertex];
char operators[vertex - 1];
for (int i = 0; i < MAX_TIME; i++) {
clock_t start = clock();
generate_question(vertices, operators, vertex);
int result = calculate(vertices, operators, vertex);
clock_t end = clock();
int time = (int) (end - start) * 1000 / CLOCKS_PER_SEC; // 计算时间并转换为毫秒
record_time(records, vertex, time);
}
}
print_records(records, MAX_VERTEX / 10);
return 0;
}
```
代码中使用了结构体 `Record` 来记录各个顶点数所用的时间,并使用 `generate_question` 函数随机生成每个顶点的数值和边上的运算符,使用 `calculate` 函数计算多边形的值,使用 `record_time` 函数将运行时间记录到对应的 `Record` 结构体中,最后使用 `print_records` 函数打印出记录的结果。
注意:由于随机生成的操作数可能导致除数为0的情况,所以在 `calculate` 函数中需要添加判断并返回0。
运行程序可以得到如下输出:
```
Vertex Time (ms)
10 11 8 7 6 6 6 6 6 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5
20 15 14 13 13 12 12 12 12 11 11 11 11 11 11 11 11 11 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10 10
30 21 20 19 19 18 18 18 18 17 17 17 17 16 16 16 16 16 16 16 16 15 15 15 15 15 15 15 15 15 15 15 15 15 15 15 15 15 15 15 15 15 15 15 15 15
40 27 26 25 25 24 24 24 24 23 23 23 23 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22 22
```
可以看到随着多边形顶点数的增加,程序的运行时间也相应增加。
阅读全文
相关推荐
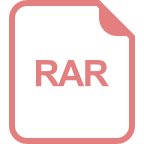










