spring boot中配置aop
时间: 2023-10-30 09:10:37 浏览: 114
在Spring Boot中配置AOP,需要遵循以下步骤:
1. 添加AOP依赖
在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
```
2. 创建切面类
在切面类中,编写需要执行的切面方法。例如,下面的切面类定义了一个方法,在执行被标注了@LogAnnotation注解的方法前后,打印日志:
```java
@Aspect
@Component
public class LogAspect {
private final Logger logger = LoggerFactory.getLogger(LogAspect.class);
@Pointcut("@annotation(com.example.demo.annotation.LogAnnotation)")
public void logPointCut() {
}
@Before("logPointCut()")
public void before(JoinPoint joinPoint) {
MethodSignature signature = (MethodSignature) joinPoint.getSignature();
Method method = signature.getMethod();
logger.info("Executing method: {}", method.getName());
}
@After("logPointCut()")
public void after(JoinPoint joinPoint) {
MethodSignature signature = (MethodSignature) joinPoint.getSignature();
Method method = signature.getMethod();
logger.info("Finished executing method: {}", method.getName());
}
}
```
上面的切面类使用@Aspect注解标注,同时也需要被@Component注解标注,以便Spring Boot能够扫描到它。
3. 配置AOP
在应用程序的配置文件(如application.properties)中,可以配置AOP的一些属性,例如开启AspectJ自动代理:
```
spring.aop.auto=true
```
这样,Spring Boot会自动为标注有@Aspect注解的切面类创建代理对象。
4. 使用AOP
在需要使用AOP的地方,使用@LogAnnotation注解标注即可:
```java
@RestController
public class HelloController {
@GetMapping("/hello")
@LogAnnotation
public String hello() {
return "Hello, World!";
}
}
```
这样,在执行hello()方法前后,就会触发LogAspect中定义的before()和after()方法,打印日志。
总结
通过上面的步骤,就可以在Spring Boot中配置AOP了。在实际开发中,我们可以使用AOP来实现日志记录、事务管理、安全检查等功能。
阅读全文
相关推荐
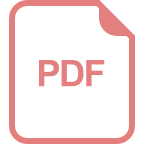
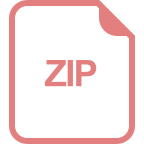
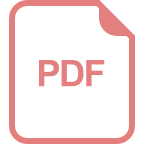
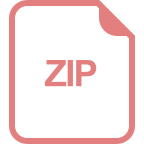
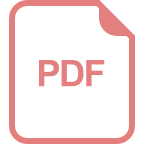
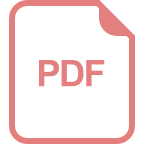
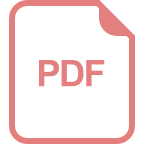
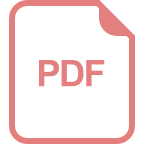
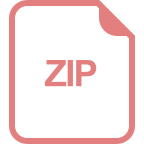
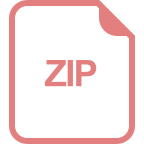
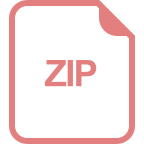
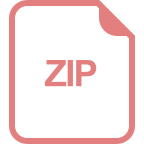
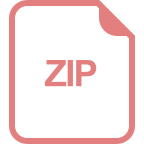
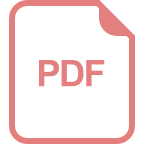
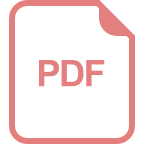