vector容器中数据存入set容器
时间: 2024-01-29 20:13:57 浏览: 26
可以通过以下步骤将vector容器中的数据存入set容器中:
1. 创建一个空的set容器对象。
```
std::set<int> mySet;
```
2. 使用std::copy函数将vector容器中的元素复制到set容器中。
```
std::vector<int> myVector = {1, 2, 3, 4, 5};
std::copy(myVector.begin(), myVector.end(), std::inserter(mySet, mySet.end()));
```
3. 现在,set容器中将包含vector容器中的所有元素。
注意:由于set容器不允许重复的元素,如果vector容器中存在重复的元素,那么只有一个元素会被存储到set容器中。
相关问题
怎么遍历删除vector容器中的数据
要遍历并删除vector容器中的数据,你可以使用迭代器进行操作。以下是一个示例代码:
```cpp
#include <iostream>
#include <vector>
int main() {
// 创建一个包含一些数据的vector容器
std::vector<int> numbers = {1, 2, 3, 4, 5};
// 创建一个迭代器指向vector的起始位置
std::vector<int>::iterator it = numbers.begin();
// 遍历vector容器
while (it != numbers.end()) {
// 判断当前元素是否需要删除
if (*it == 3) {
// 删除当前元素,并更新迭代器
it = numbers.erase(it);
} else {
// 更新迭代器,指向下一个元素
++it;
}
}
// 输出删除后的vector容器
for (int num : numbers) {
std::cout << num << " ";
}
std::cout << std::endl;
return 0;
}
```
在上面的代码中,我们使用迭代器`it`遍历vector容器中的元素。如果当前元素满足需要删除的条件(比如等于3),我们使用`erase`函数删除该元素,并且`erase`函数会返回指向下一个元素的迭代器。如果当前元素不需要删除,则直接更新迭代器指向下一个元素。最后,我们输出删除后的vector容器。
请注意,删除元素后,原先的迭代器会变为无效迭代器,所以需要用`erase`函数返回的迭代器来更新迭代器的值。
创建vector容器存储数据
好的,下面是创建vector容器并存储数据的示例代码:
```
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> myVector; // 创建空的vector容器
myVector.push_back(10); // 向容器中添加一个元素
myVector.push_back(20); // 向容器中添加一个元素
myVector.push_back(30); // 向容器中添加一个元素
cout << "容器中的元素:" << endl;
// 使用迭代器遍历容器中的元素并输出
for (vector<int>::iterator it = myVector.begin(); it != myVector.end(); ++it) {
cout << *it << endl;
}
return 0;
}
```
运行结果:
```
容器中的元素:
10
20
30
```
上述代码中,我们首先创建了一个空的vector容器,然后使用`push_back()`函数向容器中添加了三个元素。最后使用迭代器遍历容器中的元素并输出。
相关推荐
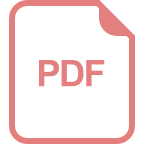












