three.js创建网格地板
时间: 2023-08-31 08:37:22 浏览: 82
### 回答1:
在 three.js 中创建网格地板,可以使用 `Mesh` 类。首先,需要创建一个网格地板的几何体,可以使用 `PlaneGeometry` 类。然后,可以使用一个材质(如 `MeshBasicMaterial` 或 `MeshLambertMaterial`)来创建网格地板的外观。最后,将几何体和材质作为参数传递给 `Mesh` 构造函数,并将新创建的网格地板添加到场景中。
例如:
```
// 创建网格地板的几何体
const geometry = new THREE.PlaneGeometry(width, height, widthSegments, heightSegments);
// 创建网格地板的材质
const material = new THREE.MeshBasicMaterial({ color: 0xffffff });
// 创建网格地板
const mesh = new THREE.Mesh(geometry, material);
// 将网格地板添加到场景中
scene.add(mesh);
```
其中,`width` 和 `height` 分别指定网格地板的宽度和高度,`widthSegments` 和 `heightSegments` 分别指定沿网格地板宽度和高度方向的网格数。
### 回答2:
在three.js中创建网格地板需要经历以下步骤:
1. 首先,我们需要导入three.js库及其他必要的依赖文件。
```
import * as THREE from 'https://cdn.skypack.dev/three@0.132.2/build/three.module.js';
```
2. 创建场景、相机和渲染器。
```
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
```
3. 创建网格地板的材质和几何体。
```
const floorGeometry = new THREE.PlaneGeometry(10, 10, 10, 10);
const floorMaterial = new THREE.MeshBasicMaterial({ color: 0x808080, wireframe: true });
```
4. 创建网格对象并将材质应用于它。
```
const floor = new THREE.Mesh(floorGeometry, floorMaterial);
scene.add(floor);
```
5. 设置相机的位置和方向。
```
camera.position.z = 5;
camera.lookAt(floor.position);
```
6. 创建动画循环函数,并在其中渲染场景。
```
function animate() {
requestAnimationFrame(animate);
renderer.render(scene, camera);
}
animate();
```
整个过程中,我们先创建了场景、相机和渲染器,然后定义了网格地板的材质和几何体。接下来,我们使用这些材质和几何体创建一个网格对象,并将它添加到场景中。然后,我们设置相机的位置和方向,以确保地板在可视范围内。最后,我们通过创建动画循环函数来持续渲染场景,使得地板可以旋转或进行其他动画效果。
这样,我们就成功地使用three.js创建了一个简单的网格地板。
相关推荐
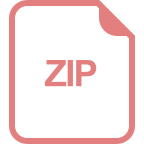
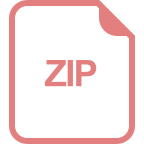














