vuex修改state
时间: 2023-07-01 22:29:17 浏览: 86
要修改Vuex中的state,需要使用mutation。mutation是Vuex中专门用于修改state的方法,它接收state作为第一个参数,接着是payload(载荷),即要修改的值。下面是一个示例:
```
// 定义mutation
const mutations = {
SET_COUNT(state, payload) {
state.count = payload
}
}
// 调用mutation
this.$store.commit('SET_COUNT', 10)
```
以上代码中,我们定义了一个名为SET_COUNT的mutation,它接收state和payload两个参数,用来修改state中的count属性。在组件中,我们可以通过调用commit方法来触发mutation,从而修改state中的值。在这个例子中,我们调用了SET_COUNT mutation,并传递了值10作为payload。这将会把state中的count属性修改为10。
相关问题
vuex修改state中的数据
要修改 Vuex 应用程序中的状态 (state),您需要提交一个 mutation。Mutation 是 Vuex 中的一种操作方式,用于更改状态。Mutation 是同步操作,且仅能通过提交 (commit) 的方式触发。下面是一个示例:
```
// 在 store 中定义 mutation
const store = new Vuex.Store({
state: {
count: 0
},
mutations: {
increment(state) {
// 变更状态
state.count++
}
}
})
// 在组件中提交 mutation
store.commit('increment')
```
在上面的示例中,`increment` 是一个 mutation,它将 `state.count` 的值加 1。要在组件中提交 mutation,只需调用 `store.commit` 并传递 mutation 的名称即可。在这个例子中,我们调用 `store.commit('increment')` 来触发 `increment` mutation。当 mutation 被触发时,它会接收当前的状态作为第一个参数。你可以通过 `state.count` 访问和修改状态。
vuex 设置state
在Vuex中设置state,需要先在store.js文件中定义一个对象,即store,它包含state、mutations、actions和getters等属性。state属性是状态对象,用于存储应用程序的所有状态,例如用户信息、页面状态等。
在定义state时,需要使用Vue提供的响应式API,即Vue.set()方法,将对象设置为响应式对象。下面是一个简单的示例:
```javascript
// store.js
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
const store = new Vuex.Store({
state: {
count: 0
},
mutations: {
increment (state) {
state.count++
}
}
})
export default store
```
在上面的示例中,state对象包含一个count属性,初始值为0。mutations属性包含一个increment方法,用于增加count的值。在mutations中,我们直接修改state对象的值,因为Vuex要求我们只能在mutations中修改state,以确保状态的可追踪性。
当我们需要使用state时,可以在组件中使用Vuex提供的辅助函数mapState将state映射到组件的计算属性中,以便在模板中使用。
```javascript
// 组件中
import { mapState } from 'vuex'
export default {
computed: {
...mapState(['count'])
},
methods: {
increment () {
this.$store.commit('increment')
}
}
}
```
在上面的示例中,我们使用mapState将store中的count属性映射到组件的计算属性中,以便在模板中使用。我们还定义了一个increment方法,当点击按钮时,调用$store.commit方法来调用increment方法,从而增加count的值。
阅读全文
相关推荐
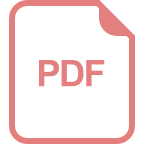
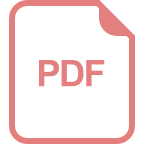
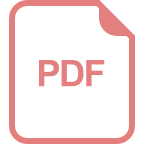

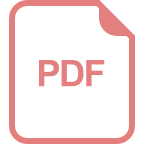
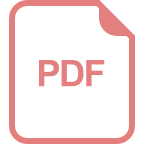







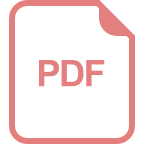

