c语言利用栈十进制转十六进制
时间: 2023-10-19 20:18:36 浏览: 158
以下是利用栈实现十进制转十六进制的C语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#define STACK_SIZE 100
typedef struct {
int top;
int data[STACK_SIZE];
} Stack;
void init(Stack *s) {
s->top = -1;
}
void push(Stack *s, int x) {
if(s->top == STACK_SIZE - 1) {
printf("Stack overflow!\n");
exit(1);
}
s->data[++(s->top)] = x;
}
int pop(Stack *s) {
if(s->top == -1) {
printf("Stack underflow!\n");
exit(1);
}
return s->data[(s->top)--];
}
int main() {
Stack s;
int num, rem, i;
printf("Enter a decimal number: ");
scanf("%d", &num);
init(&s);
while(num > 0) {
rem = num % 16;
push(&s, rem);
num /= 16;
}
printf("Hexadecimal equivalent is: ");
for(i = s.top; i >= 0; i--) {
switch(s.data[i]) {
case 10:
printf("A");
break;
case 11:
printf("B");
break;
case 12:
printf("C");
break;
case 13:
printf("D");
break;
case 14:
printf("E");
break;
case 15:
printf("F");
break;
default:
printf("%d", s.data[i]);
}
}
printf("\n");
return 0;
}
```
程序中定义了一个栈结构体,并实现了栈的初始化、入栈、出栈等操作。然后,通过循环将十进制数转换为十六进制数,并将每一位数压入栈中。最后,从栈顶开始依次弹出每一位数,并将其转换为十六进制中的字母或数字输出即可。
阅读全文
相关推荐







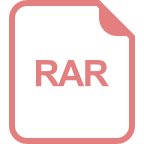








