用Java换一种更好的写法
时间: 2024-03-08 22:45:18 浏览: 15
以下是使用JavaFX编写的更优雅的代码:
```java
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.GridPane;
import javafx.scene.layout.VBox;
import javafx.scene.text.Font;
import javafx.scene.text.TextAlignment;
import javafx.stage.Stage;
public class Login extends Application {
private TextField usernameField;
private PasswordField passwordField;
private Label infoLabel;
@Override
public void start(Stage primaryStage) {
// 设置主界面标题
primaryStage.setTitle("用户登录");
// 创建用户名输入框和标签
Label usernameLabel = new Label("用户名:");
usernameField = new TextField();
usernameField.setPromptText("请输入用户名");
// 创建密码输入框和标签
Label passwordLabel = new Label("密 码:");
passwordField = new PasswordField();
passwordField.setPromptText("请输入密码");
// 创建登录按钮和取消按钮
Button loginButton = new Button("登录");
Button cancelButton = new Button("取消");
// 创建信息提示标签
infoLabel = new Label();
infoLabel.setTextAlignment(TextAlignment.CENTER);
// 设置字体和对齐方式
Font font = new Font("SimSun", 20);
usernameLabel.setFont(font);
passwordLabel.setFont(font);
loginButton.setFont(font);
cancelButton.setFont(font);
infoLabel.setFont(font);
// 创建网格布局,并将组件添加到网格中
GridPane gridPane = new GridPane();
gridPane.setAlignment(Pos.CENTER);
gridPane.setHgap(10);
gridPane.setVgap(10);
gridPane.add(usernameLabel, 0, 0);
gridPane.add(usernameField, 1, 0);
gridPane.add(passwordLabel, 0, 1);
gridPane.add(passwordField, 1, 1);
gridPane.add(loginButton, 0, 2);
gridPane.add(cancelButton, 1, 2);
gridPane.add(infoLabel, 0, 3, 2, 1);
gridPane.setPadding(new Insets(10));
// 创建垂直布局,并将网格布局添加到其中
VBox vbox = new VBox();
vbox.setAlignment(Pos.CENTER);
vbox.getChildren().add(gridPane);
// 创建场景并设置主界面大小
Scene scene = new Scene(vbox, 300, 200);
// 设置登录按钮和取消按钮的事件处理器
loginButton.setOnAction(event -> login());
cancelButton.setOnAction(event -> cancel());
// 显示主界面
primaryStage.setScene(scene);
primaryStage.show();
}
/**
* 登录事件处理器
*/
private void login() {
String username = usernameField.getText().trim();
String password = passwordField.getText().trim();
if (username.isEmpty() || password.isEmpty()) {
infoLabel.setText("请输入用户名和密码");
} else {
infoLabel.setText("用户名: " + username + " 密码: " + password);
}
}
/**
* 取消事件处理器
*/
private void cancel() {
usernameField.setText("");
passwordField.setText("");
infoLabel.setText("");
}
public static void main(String[] args) {
launch(args);
}
}
```
使用JavaFX可以更方便地创建GUI界面,代码更加简洁易读。在这个例子中,我们使用GridPane布局来排列组件,并使用VBox布局将GridPane包装起来。我们还可以使用Lambda表达式来设置按钮的事件处理器,这使得代码更加简洁。
相关推荐
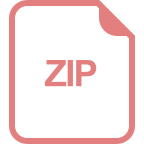
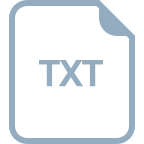
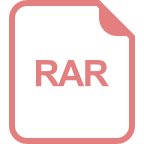














