python操作mongodb
时间: 2023-07-05 17:34:25 浏览: 72
Python 操作 MongoDB 需要安装 pymongo 库,可以使用 pip 命令进行安装。
安装 pymongo 库:
```
pip install pymongo
```
连接 MongoDB 数据库:
```python
import pymongo
client = pymongo.MongoClient("mongodb://localhost:27017/")
# 创建数据库
db = client["mydatabase"]
```
查看 MongoDB 数据库:
```python
print(client.list_database_names())
```
创建集合:
```python
# 在 mydatabase 数据库中创建一个名为 "customers" 的集合
customers = db["customers"]
```
插入文档:
```python
# 在 customers 集合中插入一条文档
mydict = { "name": "John", "address": "Highway 37" }
x = customers.insert_one(mydict)
```
查找文档:
```python
# 查找 customers 集合中所有文档
for x in customers.find():
print(x)
```
更新文档:
```python
# 将 customers 集合中所有地址为 "Highway 37" 的文档更新为 "Park Lane 38"
myquery = { "address": "Highway 37" }
newvalues = { "$set": { "address": "Park Lane 38" } }
customers.update_many(myquery, newvalues)
```
删除文档:
```python
# 删除 customers 集合中地址为 "Park Lane 38" 的文档
myquery = { "address": "Park Lane 38" }
customers.delete_one(myquery)
```
相关推荐
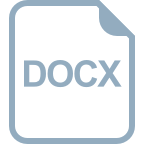
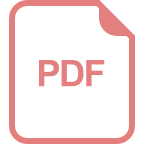
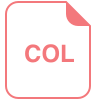
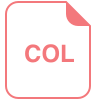
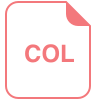
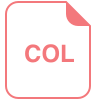
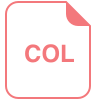









