springboot使用jdbc执行sql查询的方法
时间: 2024-05-13 08:16:06 浏览: 123
SpringBoot使用JDBC执行SQL查询可以通过以下步骤实现:
1. 配置数据源,包括数据库连接信息、用户名、密码等。
2. 创建 JdbcTemplate 对象,它可以通过数据源执行 SQL 语句。
3. 调用 JdbcTemplate 的 query 方法执行查询语句,并通过 RowMapper 对查询结果进行转换。
以下是一段示例代码:
```java
// 配置数据源
@Bean
public DataSource dataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
dataSource.setDriverClassName("com.mysql.cj.jdbc.Driver");
dataSource.setUrl("jdbc:mysql://localhost:3306/test");
dataSource.setUsername("root");
dataSource.setPassword("123456");
return dataSource;
}
// 创建 JdbcTemplate 对象
@Bean
public JdbcTemplate jdbcTemplate() {
return new JdbcTemplate(dataSource());
}
// 执行查询语句
String sql = "SELECT name, age FROM user WHERE id = ?";
Object[] params = new Object[] {1};
User user = jdbcTemplate.queryForObject(sql, params, new RowMapper<User>() {
public User mapRow(ResultSet rs, int rowNum) throws SQLException {
User user = new User();
user.setName(rs.getString("name"));
user.setAge(rs.getInt("age"));
return user;
}
});
```
以上示例代码演示了如何使用 SpringBoot 和 JDBC 执行 SQL 查询语句。其中,我们通过注入数据源对象和 JdbcTemplate 对象实现了操作数据库的功能,通过 RowMapper 对查询结果进行转换。
阅读全文
相关推荐
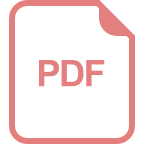
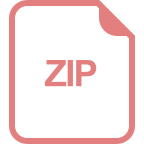

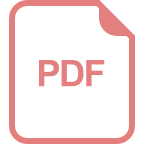
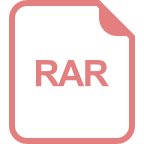
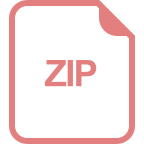
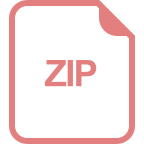
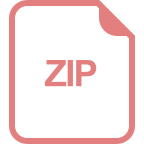
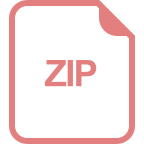
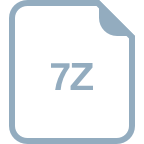
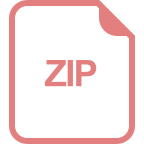
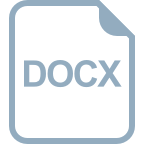
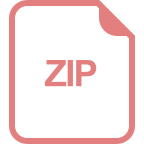
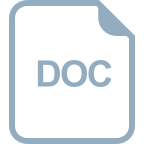

