matble编程实现:读入并显示不同类型的图像文件。
时间: 2024-09-23 10:16:44 浏览: 47
Matplotlib是一个强大的数据可视化库,在Python中常用于创建静态、动态、交互式的数据图形。如果你想用它读取并显示不同类型的图像文件,可以借助其`imshow`函数。以下是基本步骤:
1. 首先,确保已经安装了matplotlib和PIL(Python Imaging Library,如果需要处理各种图像格式)。如果没有,你可以通过pip进行安装:
```
pip install matplotlib pillow
```
2. 导入必要的模块:
```python
import matplotlib.pyplot as plt
from PIL import Image
```
3. 使用PIL的`Image.open()`函数打开图像文件,支持多种格式:
```python
image = Image.open('image_file.jpg') # 替换为你想处理的文件路径和名称
```
4. 转换为适合matplotlib显示的数组(对于彩色图像通常是RGB模式):
```python
image_array = np.array(image)
```
5. 创建一个新的图形窗口,并显示图像:
```python
fig, ax = plt.subplots()
ax.imshow(image_array)
plt.show()
```
如果你有多个不同类型的图像想要展示,可以将上述代码放在循环里分别读取。
相关问题
自己编写matble函数实现线性预测谱的绘制
以下是一个示例代码,其中使用了matlab自带的fft函数进行频谱分析,并将结果用plot函数绘制出来。
```matlab
function lpc_spectrogram(signal, Fs, order, windowSize, overlap)
% signal: 输入信号
% Fs: 采样频率
% order: LPC预测的阶数
% windowsSize: 窗口大小
% overlap: 帧之间的重叠比例
% 设置分析参数
N = length(signal); % 信号长度
w = hamming(windowSize); % 窗函数
step = windowSize - overlap * windowSize; % 帧移
numFrames = floor((N - windowSize) / step) + 1; % 分帧数
% 初始化输出数据
spec = zeros(windowSize, numFrames); % 频谱图矩阵
% 分帧处理
for i = 1:numFrames
startIdx = (i - 1) * step + 1; % 起始位置
endIdx = startIdx + windowSize - 1; % 结束位置
x = signal(startIdx:endIdx) .* w; % 加窗
a = lpc(x, order); % LPC分析
[H, f] = freqz(1, a, windowSize, Fs); % 频谱分析
spec(:, i) = abs(H); % 取模值
end
% 绘制频谱图
freq = linspace(0, Fs / 2, windowSize / 2 + 1); % 频率轴
time = linspace(0, N / Fs, numFrames); % 时间轴
imagesc(time, freq, 20 * log10(spec(1:windowSize / 2 + 1, :))); % 绘制频谱图
axis xy; colormap(jet); colorbar; % 设置坐标轴、色标
xlabel('Time [s]'); ylabel('Frequency [Hz]');
title('Linear Predictive Coding Spectrogram');
end
```
使用示例:
```matlab
load('example_signal.mat'); % 载入样例信号
Fs = 16000; % 采样频率
order = 16; % 预测阶数
windowSize = 512; % 窗口大小
overlap = 0.75; % 帧重叠比例
lpc_spectrogram(signal, Fs, order, windowSize, overlap); % 绘制频谱图
```
直方图均衡化与matble代码实现术之多
直方图均衡化是一种图像增强技术,它可以将图像的灰度值拉伸到整个灰度级范围内,从而提高图像的对比度和细节。下面是用Matlab实现直方图均衡化的步骤:
1. 读入图像并转化为灰度图像
```
img = imread('lena.jpg');
gray_img = rgb2gray(img);
```
2. 计算灰度直方图
```
[h, x] = imhist(gray_img);
```
3. 计算累积分布函数CDF
```
cdf = cumsum(h) / numel(gray_img);
```
4. 将CDF映射到0~255的灰度级范围内
```
map = uint8(255 * cdf);
```
5. 应用映射函数,得到均衡化后的图像
```
eq_img = map(gray_img + 1);
```
6. 显示原图和均衡化后的图像
```
subplot(1,2,1)
imshow(gray_img)
title('Original Image')
subplot(1,2,2)
imshow(eq_img)
title('Equalized Image')
```
以上就是用Matlab实现直方图均衡化的步骤。
阅读全文
相关推荐
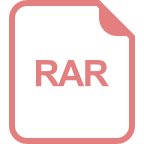
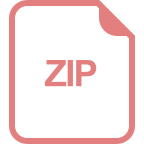
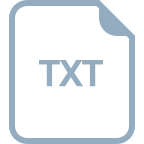
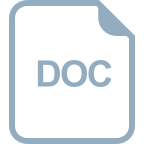






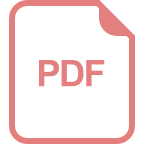
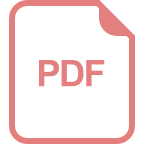