c语言函数题求中位数float fun
时间: 2024-12-24 21:13:44 浏览: 4
在C语言中,如果你想要编写一个函数来计算一组浮点数的中位数,你可以采用排序数组元素然后取中间值的方式来实现。由于C语言标准库并未直接提供排序数组的功能,所以通常会借助其他算法如快速排序、冒泡排序或使用stdlib.h中的qsort()函数来进行排序。
这是一个简单的示例函数,假设输入数组已经包含并且长度大于0:
```c
#include <stdio.h>
#include <stdlib.h>
// 自定义比较函数用于排序
int compare(const void *a, const void *b) {
return (*(const float *)a - *(const float *)b);
}
// 函数来计算中位数
float median(float arr[], int n) {
// 排序数组
qsort(arr, n, sizeof(float), compare);
// 计算中位数
if (n % 2 == 0) { // 如果数组长度偶数,取中间两个数的平均值
int mid = n / 2;
return (arr[mid - 1] + arr[mid]) / 2.0;
} else { // 如果数组长度奇数,取中间的那个数
int mid = n / 2;
return arr[mid];
}
}
int main() {
float numbers[] = {5.5, 2.3, 9.7, 4.1, 6.8};
int size = sizeof(numbers) / sizeof(numbers[0]);
printf("The median of the array is: %.2f\n", median(numbers, size));
return 0;
}
```
阅读全文
相关推荐
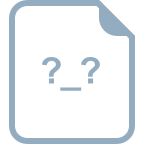
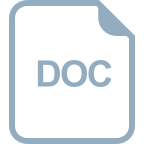
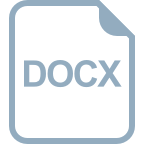
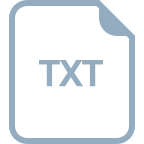
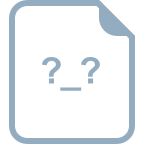
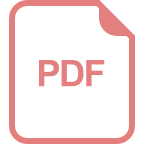
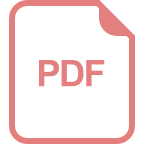
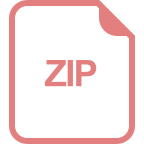
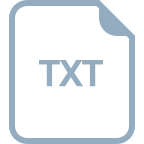
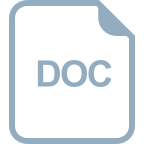
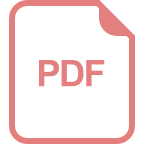
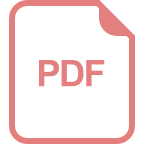
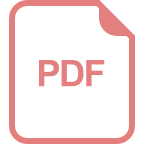
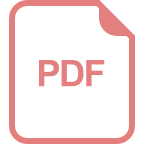
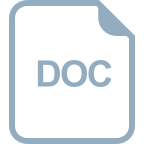
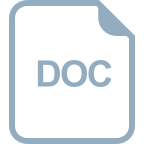
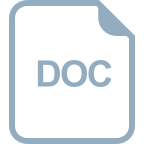
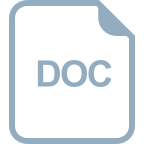
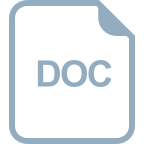