请写出包含头的完整的代码
时间: 2024-09-19 08:04:02 浏览: 22
当然,这里是完整的C++代码,包含了创建链表、遍历链表以及按位查找的示例。请注意,这个例子中并没有实际实现按位查找,因为它不适合链表结构,链表通常是顺序访问的,而非逐位访问。以下是整个程序的框架:
```cpp
#include <iostream>
// 定义链表节点结构体
struct Node {
int data;
Node* next;
};
// 创建链表
Node* createLinkedList() {
Node* head = new Node();
for (size_t i = 1; i <= 9; ++i) {
Node* newNode = new Node();
newNode->data = i;
newNode->next = nullptr;
if (!head->next) {
head->next = newNode;
} else {
Node* current = head->next;
while (current->next) {
current = current->next;
}
current->next = newNode;
}
}
return head;
}
// 遍历并打印链表
void printList(Node* node) {
while (node != nullptr) {
std::cout << node->data << " ";
node = node->next;
}
std::cout << std::endl;
}
// 指定位置按位查找,这里只做示例,实际上链表不适合按位查找
bool isBitSet(int num, int bitPosition) {
return num & (1 << bitPosition); // 这里仅适用于整数的一位操作
}
int main() {
// 创建链表
Node* head = createLinkedList();
// 打印链表
std::cout << "链表元素: ";
printList(head);
// 示例按位查找
int elementToSearch = 10; // 假设要搜索的值
int bitPositionToCheck = 3; // 假设要查看的位数
bool bitFound = isBitSet(elementToSearch, bitPositionToCheck);
std::cout << "第" << bitPositionToCheck << "位在元素" << elementToSearch << "上是否为1: " << bitFound << std::endl;
// 清理内存,防止内存泄漏
Node* temp = head;
while (temp) {
Node* nextTemp = temp->next;
delete temp;
temp = nextTemp;
}
return 0;
}
相关推荐
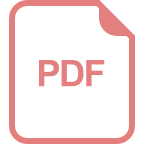
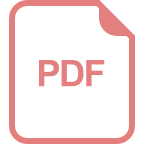
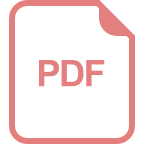














