python读取excel某列内容制作词云图并用条形图统计词频的代码
时间: 2024-10-18 07:12:26 浏览: 38
在Python中,你可以使用`pandas`库读取Excel文件,`jieba`库处理中文分词,然后结合`wordcloud`和`matplotlib`库生成词云图和条形图。以下是一个简单的示例:
```python
# 导入所需库
import pandas as pd
from wordcloud import WordCloud
import matplotlib.pyplot as plt
import jieba
# 读取Excel文件
df = pd.read_excel('your_file.xlsx') # 将 'your_file.xlsx' 替换为你的Excel文件路径及名称
# 选定你要分析的列
column_name = 'Column_Name' # 将 'Column_Name' 替换为你想分析的具体列名
text_data = df[column_name].values.tolist()
# 对文本数据进行分词
all_words = []
for text in text_data:
words = jieba.lcut(text)
all_words.extend(words)
# 生成词频统计
word_freq = {}
for word in all_words:
if word not in word_freq:
word_freq[word] = 0
word_freq[word] += 1
# 按词频排序
sorted_word_freq = sorted(word_freq.items(), key=lambda x: x[1], reverse=True)
# 生成词云图
wc = WordCloud(width=800, height=400, background_color='white', min_font_size=1).generate_from_frequencies(sorted_word_freq)
plt.imshow(wc, interpolation="bilinear")
plt.axis("off")
plt.show()
# 生成条形图
plt.figure(figsize=(10, 6))
plt.bar(sorted_word_freq.keys(), [freq for freq, _ in sorted_word_freq])
plt.title('Word Frequency')
plt.xlabel('Words')
plt.ylabel('Frequency')
plt.show()
```
在这个代码中,你需要替换文件路径、列名以及可能需要调整的词云和条形图参数。运行完这个脚本后,你会得到一个展示数据中常见词汇的词云图和按频率排序的条形图。
阅读全文
相关推荐
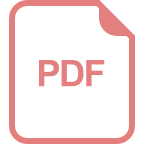
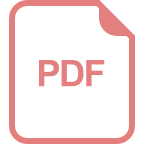
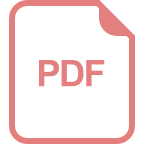
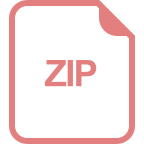
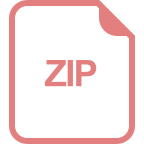
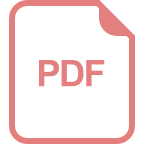
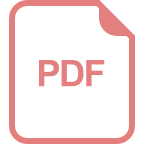
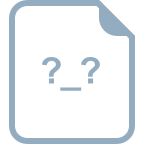
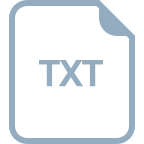
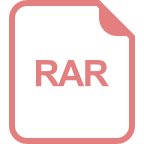
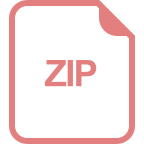
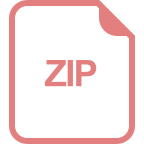
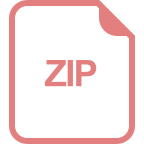
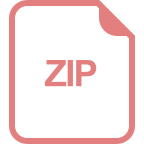