opencv填充多边形
时间: 2023-09-13 13:09:22 浏览: 54
要使用OpenCV来填充多边形,可以按照以下步骤进行操作:
1. 导入必要的库:
```python
import cv2
import numpy as np
```
2. 创建一个空白图像:
```python
image = np.zeros((height, width, 3), dtype=np.uint8)
```
这里的 `height` 和 `width` 是图像的高度和宽度。
3. 定义多边形的顶点坐标:
```python
pts = np.array([[x1, y1], [x2, y2], [x3, y3], ...], np.int32)
```
`x1, y1, x2, y2, ...` 是多边形的顶点坐标。
4. 将多边形填充到图像中:
```python
cv2.fillPoly(image, [pts], (b, g, r))
```
`b, g, r` 是填充的颜色,由蓝色、绿色和红色通道值组成。
完整的代码示例:
```python
import cv2
import numpy as np
# 创建空白图像
height, width = 500, 500
image = np.zeros((height, width, 3), dtype=np.uint8)
# 定义多边形的顶点坐标
pts = np.array([[100, 100], [200, 50], [300, 200], [150, 300]], np.int32)
# 将多边形填充到图像中
cv2.fillPoly(image, [pts], (255, 0, 0))
# 显示图像
cv2.imshow("Filled Polygon", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这将创建一个大小为 500x500 的空白图像,并在其中填充一个多边形。多边形的顶点坐标定义在 `pts` 中,颜色为蓝色。运行代码后,将显示填充了多边形的图像。
相关推荐
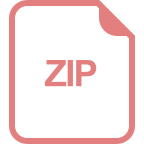
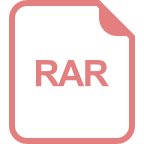














