c++实现SM4算法的编程代码
时间: 2024-07-28 15:00:25 浏览: 72
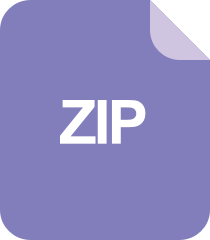
使用C++语言实现的SM2国密算法,运用tommath库来进行公私钥的计算
SM4(Secure Message Block Chaining Message Authentication Code)算法是基于国际标准ISO/IEC 18033-3实现的消息认证码算法,用于保护数据传输的安全性。在C++中实现SM4算法,你需要了解基本的数据结构和函数,因为SM4涉及到位操作、字节处理等。
以下是一个简化的SM4算法实现示例,仅提供基本的结构,实际使用时需要考虑错误处理、加密和解密流程,并且可能需要包含更完整的类型定义和其他辅助函数:
```cpp
#include <cstdint>
// SM4 key和block size
const uint32_t SM4_KEY_SIZE = 32;
const uint32_t SM4_BLOCK_SIZE = 16;
class SM4 {
private:
uint32_t key; // SM4密钥数组
public:
void setKey(const uint8_t* keyData, size_t keyLength) {
if (keyLength != SM4_KEY_SIZE) {
throw std::invalid_argument("Invalid key length");
}
for (size_t i = 0; i < SM4_KEY_SIZE; ++i) {
key[i / 4][i % 4] = keyData[i];
}
}
// Simplified implementation of SM4 encryption/decryption steps
uint8_t* encryptBlock(uint8_t* input, uint8_t* output) {
// ... (implement SBox, rounds, etc.)
return output;
}
uint8_t* decryptBlock(uint8_t* input, uint8_t* output) {
// ... (reverse the encryption steps)
return output;
}
};
// 示例用法:
int main() {
SM4 sm4;
uint8_t keyData[SM4_KEY_SIZE] = { /* your 32-byte key here */ };
sm4.setKey(keyData, SM4_KEY_SIZE);
// Encrypt and Decrypt blocks using sm4.encryptBlock() and sm4.decryptBlock()
// ...
return 0;
}
```
请注意,这个代码片段仅作为概念示例,并未包含完整的SM4算法细节。实际使用时,你需要从官方文档或可靠的第三方库中获取完整的SM4算法实现。
阅读全文
相关推荐
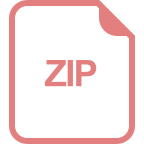
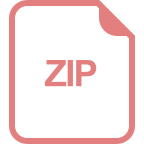
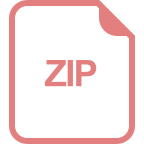
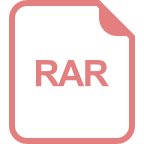
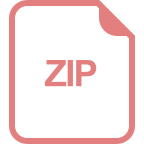
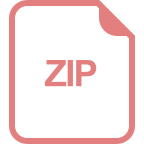
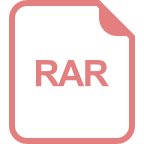
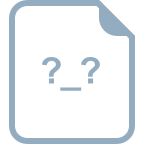
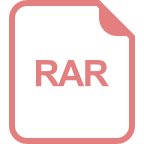
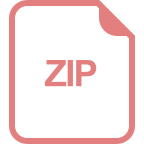
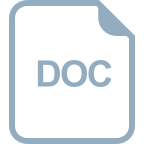
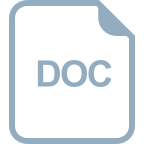
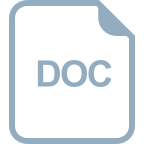
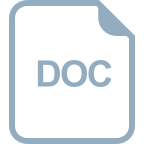
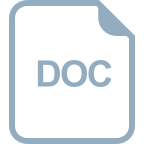
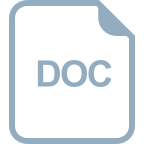

