用c++实现对双向链表的增删改查,数据包括,姓名,学号,并要求输出所有数据,并且使用头插法,和尾插法
时间: 2024-09-23 13:13:13 浏览: 33
在C++中,实现一个双向链表(Doubly Linked List)来存储学生的信息(姓名和学号),可以按照以下步骤进行:
首先,我们需要定义一个Node结构体,包含数据成员(姓名和学号)以及指向前驱(prev)和后继(next)节点的指针。
```cpp
struct Student {
std::string name;
int id;
struct Node* prev;
struct Node* next;
};
struct Node {
Student data;
Node* prev;
Node* next;
};
```
接下来,我们可以创建链表相关的函数:
1. **构造函数和析构函数**:用于初始化和销毁链表。
```cpp
Node* createNode(Student student) {
// 创建一个新的节点并分配内存
}
~StudentList() {
clearList(); // 清理链表
}
void clearList() {
// 遍历链表删除每个节点
}
```
2. **插入操作**:包括头插法(insertAtHead)和尾插法(insertAtTail)。
```cpp
void insertAtHead(Student student) {
Node* newNode = createNode(student);
if (!head) {
head = newNode;
} else {
newNode->next = head;
head->prev = newNode;
head = newNode;
}
}
void insertAtTail(Student student) {
Node* newNode = createNode(student);
if (!tail) {
tail = newNode;
} else {
tail->next = newNode;
newNode->prev = tail;
tail = newNode;
}
}
```
3. **查找、修改和删除**:
- 查找特定学生:searchById(int id)
- 修改学生信息:updateDataByIndex(int index, Student newData)
- 删除学生:deleteStudentByName(std::string name)
4. **遍历和打印所有数据**:
```cpp
void printAllStudents() {
if (head) {
Node* temp = head;
while (temp != nullptr) {
std::cout << "Name: " << temp->data.name << ", ID: " << temp->data.id << std::endl;
temp = temp->next;
}
} else {
std::cout << "No students in the list." << std::endl;
}
}
```
阅读全文
相关推荐
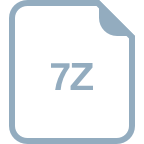
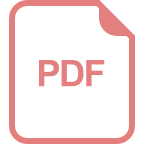
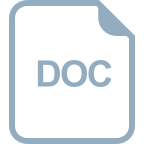
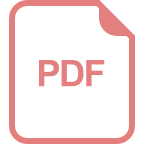
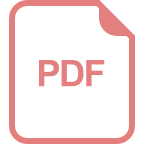
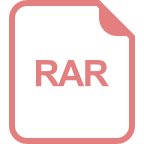
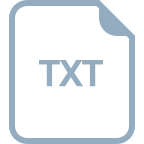
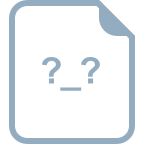
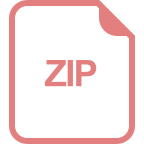
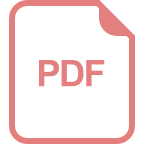
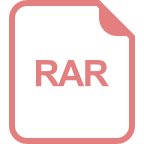
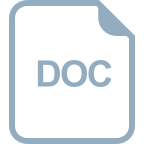
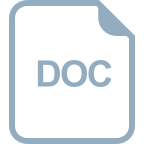
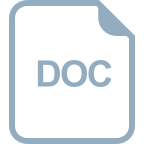
