请用C语言编写一个程序,给一个链表,删除链表的倒数第i个节点,并返回链表头节点,不要出现typedef struce
时间: 2024-09-18 21:18:57 浏览: 42
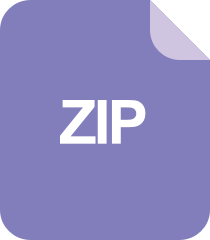
c语言-c语言编程基础之leetcode题解第19题删除链表的倒数第N个结点.zip
在C语言中,我们可以使用指针和链表的基本操作来实现这个功能。假设链表的结构体定义如下:
```c
struct Node {
int data;
struct Node* next;
};
```
这里是一个示例函数,它接受链表的头节点指针,以及要删除的倒数第i个节点的位置i:
```c
struct Node* deleteLastButIth(struct Node* head, int i) {
if (head == NULL || i <= 0) return head; // 链表为空或i小于等于0,直接返回
struct Node* current = head;
int count = 1; // 当前遍历到的节点计数
// 找到倒数第i个节点
while (current->next != NULL && count < i) {
current = current->next;
count++;
}
// 如果count小于i,说明i超出链表长度,直接返回原头节点
if (count < i) return head;
// 删除倒数第i个节点
if (current->next != NULL) {
current->next = current->next->next;
} else {
// 如果要删除的是最后一个节点
head = head->next;
}
return head;
}
```
注意,这个函数假设i是非负整数并且链表至少有i+1个节点。如果链表非常长,这种方法可能会消耗较多内存,因为我们需要遍历整个链表。
阅读全文
相关推荐
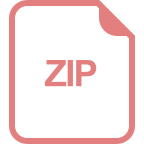
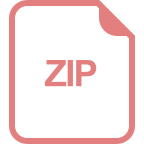








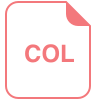
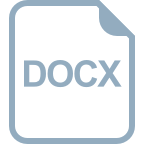
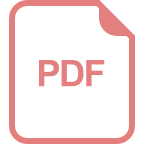
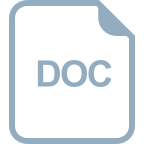


