vue项目pc端自适应
时间: 2023-08-17 12:06:11 浏览: 145
对于Vue项目在PC端的自适应,你可以使用CSS的媒体查询来实现页面的响应式布局。下面是一些基本的步骤:
1. 在项目中的CSS文件中,定义不同屏幕尺寸下的样式。可以使用@media规则来设置媒体查询。
例如,你可以在CSS文件中添加以下代码:
```css
/* 默认样式 */
/* 在宽度小于等于768px时应用的样式 */
@media (max-width: 768px) {
/* 设置适应小屏幕的样式 */
}
/* 在宽度大于768px但小于等于1024px时应用的样式 */
@media (min-width: 769px) and (max-width: 1024px) {
/* 设置适应中等屏幕的样式 */
}
/* 在宽度大于1024px时应用的样式 */
@media (min-width: 1025px) {
/* 设置适应大屏幕的样式 */
}
```
2. 在Vue组件中引入CSS文件。
可以使用`<style>`标签将CSS样式直接写在Vue组件中,或者在Vue组件中引入外部的CSS文件。
例如,在Vue组件中引入外部CSS文件:
```vue
<template>
<!-- 组件内容 -->
</template>
<script>
export default {
// 组件逻辑
}
</script>
<style src="./your-styles.css"></style>
```
3. 在Vue组件中使用class绑定来动态切换不同的样式。
你可以在Vue组件中使用`v-bind:class`指令来动态地切换不同的样式。
例如,在Vue组件的模板中添加以下代码:
```vue
<template>
<div :class="['container', { 'small-screen': isSmallScreen, 'medium-screen': isMediumScreen, 'large-screen': isLargeScreen }]">
<!-- 页面内容 -->
</div>
</template>
<script>
export default {
data() {
return {
isSmallScreen: false,
isMediumScreen: false,
isLargeScreen: false
}
},
mounted() {
// 在组件挂载后,根据屏幕宽度设置相应的布尔值
this.updateScreenSize()
window.addEventListener('resize', this.updateScreenSize)
},
destroyed() {
window.removeEventListener('resize', this.updateScreenSize)
},
methods: {
updateScreenSize() {
const screenWidth = window.innerWidth
this.isSmallScreen = screenWidth <= 768
this.isMediumScreen = screenWidth > 768 && screenWidth <= 1024
this.isLargeScreen = screenWidth > 1024
}
}
}
</script>
<style>
/* 添加不同屏幕尺寸下的样式 */
.container {
/* 默认样式 */
}
.small-screen {
/* 适应小屏幕的样式 */
}
.medium-screen {
/* 适应中等屏幕的样式 */
}
.large-screen {
/* 适应大屏幕的样式 */
}
</style>
```
通过上述方法,你可以根据不同的屏幕尺寸来设置不同的样式,从而实现Vue项目在PC端的自适应。
阅读全文
相关推荐
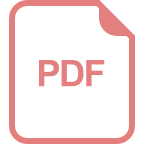
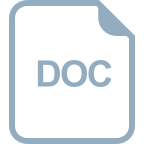




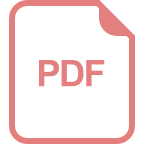
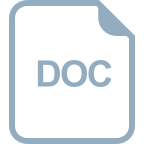








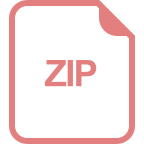