mongoose populate和populated
时间: 2024-06-06 20:05:58 浏览: 174
`populate()`是mongoose提供的一个方法,用来在查询结果中填充指定字段的关联数据。它可以方便地将两个或多个集合中的数据关联起来,从而避免了多次查询数据库的步骤,提高了查询效率。`populate()`方法接受一个参数,参数是一个字符串或一个对象,用于指定要填充的字段和相关联的集合。
`populated`是一个mongoose查询结果中的属性,它用于获取填充后的关联数据。当使用`populate()`方法填充一个或多个字段时,查询结果中对应的字段会变成一个填充后的对象,该对象有一个`populated`属性,该属性就是填充后的关联数据。`populated`属性的值可以是一个单个文档对象,也可以是一个文档对象数组,具体取决于原始查询结果中对应字段的数据类型。
相关问题
mongoose populate
Mongoose's `populate()` function is used to populate referenced fields in a document with actual data from another collection. It allows you to perform database joins in MongoDB.
Let's say you have two models: `User` and `Post`. The `Post` model has a reference to the `User` model through a field called `author`.
To use `populate()`, you would first define your models using Mongoose:
```javascript
const mongoose = require('mongoose');
const userSchema = new mongoose.Schema({
name: String,
age: Number,
});
const postSchema = new mongoose.Schema({
title: String,
content: String,
author: {
type: mongoose.Schema.Types.ObjectId,
ref: 'User',
},
});
const User = mongoose.model('User', userSchema);
const Post = mongoose.model('Post', postSchema);
```
Now, let's say you want to find all posts and populate the `author` field with the actual user data. You can do that using the `populate()` function:
```javascript
Post.find().populate('author').exec((err, posts) => {
if (err) {
console.error(err);
return;
}
console.log(posts);
});
```
This will fetch all posts and populate the `author` field with the corresponding user data from the `User` collection. You can then access the populated data using dot notation, like `post.author.name`.
You can also populate multiple fields by passing an array of field names to the `populate()` function, like `populate(['author', 'category'])`.
It's important to note that populate is an expensive operation, as it involves multiple database queries. So, use it judiciously and consider using it only when necessary to avoid performance issues.
mongoose中 populate怎么用
在 Mongoose 中,populate 方法可以用来填充一个或多个字段的引用。当我们在一个模型中引用了另一个模型的某些属性时,我们可以使用 populate 方法来填充这些属性,以便在查询时一并返回所引用的文档。
具体使用方法如下:
1. 在定义 schema 时,使用 ref 字段来指定所引用的模型,例如:
```
const userSchema = new mongoose.Schema({
name: String,
email: String
});
const postSchema = new mongoose.Schema({
title: String,
content: String,
author: {
type: mongoose.Schema.Types.ObjectId,
ref: 'User'
}
});
```
在这个例子中,postSchema 引用了 User 模型中的 _id 属性,使用 ref 字段来指定所引用的模型为 User。
2. 在查询时使用 populate 方法来填充所引用的文档,例如:
```
Post.find().populate('author').exec((err, posts) => {
// 处理查询结果
});
```
在这个例子中,我们使用 populate 方法来填充 author 字段引用的 User 模型对应的文档。这样,在查询结果中,每个 post 文档的 author 字段将会被替换为对应的 User 文档。
除了填充单个字段,我们还可以填充多个字段,例如:
```
Post.find().populate('author').populate('comments').exec((err, posts) => {
// 处理查询结果
});
```
这个例子中,我们首先使用 populate 方法来填充 author 字段,然后又使用 populate 方法来填充 comments 字段。这样,在查询结果中,每个 post 文档的 author 字段和 comments 字段都将会被替换为对应的文档。
阅读全文
相关推荐
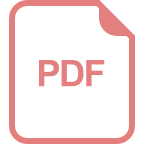
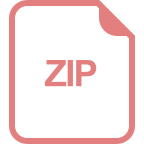
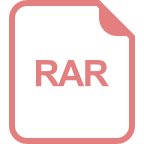













