linux线程倒计时
时间: 2024-09-26 14:07:32 浏览: 29
在Linux中,可以使用互斥锁(Mutex)和条件变量(Condition Variable,通常简称为CondVar)配合来实现线程间的倒计时。这里是一个简单的例子,假设有一个主线程启动若干个工作线程,并需要在某个时间点让它们停止工作:
```c
#include <pthread.h>
#include <unistd.h>
#include <time.h>
// 创建互斥锁和条件变量
pthread_mutex_t mutex;
pthread_cond_t cond;
void *thread_func(void *arg) {
pthread_mutex_lock(&mutex);
while (counter > 0) { // counter表示倒计时数值
pthread_cond_wait(&cond, &mutex); // 线程进入等待状态
printf("Worker thread: %lu seconds left.\n", counter);
}
pthread_mutex_unlock(&mutex);
printf("Worker thread exiting...\n");
}
int main() {
int counter = 10; // 倒计时10秒
pthread_t worker_threads[10]; // 假设有10个工作线程
// 创建并启动工作线程
for (int i = 0; i < 10; ++i) {
if (pthread_create(&worker_threads[i], NULL, thread_func, NULL)) {
perror("Error creating thread");
return -1;
}
}
// 主线程开始倒计时,然后唤醒所有工作线程
for (int i = 0; i < 10; ++i) {
sleep(1); // 每秒减少一次计数
counter--;
pthread_mutex_lock(&mutex);
if (counter == 0) {
pthread_cond_signal(&cond); // 唤醒等待的所有线程
} else {
pthread_cond_wait(&cond, &mutex); // 自己也进入等待,直到计数到0
}
pthread_mutex_unlock(&mutex);
}
// 主线程结束,解锁全局资源
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
// 等待所有线程完成
for (int i = 0; i < 10; ++i) {
pthread_join(worker_threads[i], NULL);
}
return 0;
}
```
在这个示例中,`main`函数负责控制倒计时,当计数到0时通过信号通知线程退出。每个工作线程会在接到信号后立即结束。
相关推荐
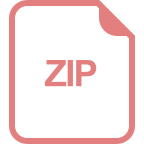
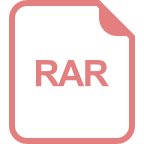














