SM3hmac的Java
时间: 2024-01-17 14:12:01 浏览: 34
SM3hmac是一种基于SM3哈希算法的HMAC(加密消息认证码)算法。在Java中实现SM3hmac可以使用Bouncy Castle库来完成。
首先,确保你已经引入了Bouncy Castle库的依赖。你可以通过Maven来添加以下依赖:
```xml
<dependency>
<groupId>org.bouncycastle</groupId>
<artifactId>bcpkix-jdk15on</artifactId>
<version>1.69</version>
</dependency>
```
然后,你可以使用以下代码来计算SM3hmac:
```java
import org.bouncycastle.crypto.digests.SM3Digest;
import org.bouncycastle.crypto.macs.HMac;
import org.bouncycastle.crypto.params.KeyParameter;
import java.nio.charset.StandardCharsets;
public class SM3HmacExample {
public static void main(String[] args) {
String message = "Hello World";
byte[] key = "SecretKey".getBytes(StandardCharsets.UTF_8);
byte[] hmac = calculateHmac(message.getBytes(StandardCharsets.UTF_8), key);
System.out.println("SM3-HMAC: " + bytesToHex(hmac));
}
public static byte[] calculateHmac(byte[] message, byte[] key) {
SM3Digest digest = new SM3Digest();
HMac hmac = new HMac(digest);
hmac.init(new KeyParameter(key));
hmac.update(message, 0, message.length);
byte[] mac = new byte[digest.getDigestSize()];
hmac.doFinal(mac, 0);
return mac;
}
public static String bytesToHex(byte[] bytes) {
StringBuilder hex = new StringBuilder();
for (byte b : bytes) {
hex.append(String.format("%02x", b));
}
return hex.toString();
}
}
```
在上面的示例中,我们使用`calculateHmac`方法来计算SM3-HMAC值,传入消息和密钥。然后,我们将HMAC值转换为十六进制字符串并打印出来。
请注意,为了演示目的,这里使用了硬编码的密钥和消息。在实际应用中,你可能需要根据具体需求进行替换。
希望以上代码对你有帮助!
相关推荐
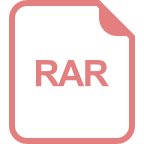














