java web实现实现一个“个人日志”系统:第一阶段实现功能:用户可注册、登录,发表个人日志(每个用户可以看到其他用户发的日志),可以对所有个人日志进行评论,可退出系统;第二阶段实现功能:用户可以修改或删除自己发布的个人日志,可以删除自己日志下的评论,可以删除自己对其他用户日志的评论(相关细节可自行根据合理性设计)的代码
时间: 2024-02-25 19:56:04 浏览: 71
好的,这里给您提供一份大致的代码实现参考,仅供参考:
1. 用户注册功能
```
@RequestMapping(value = "/register", method = RequestMethod.POST)
public String register(User user, Model model) {
if (userService.createUser(user)) {
model.addAttribute("msg", "注册成功,请登录!");
return "login";
} else {
model.addAttribute("msg", "注册失败,请重试!");
return "register";
}
}
```
2. 用户登录功能
```
@RequestMapping(value = "/login", method = RequestMethod.POST)
public String login(User user, HttpSession session, Model model) {
User loginUser = userService.checkUser(user);
if (loginUser != null) {
session.setAttribute("user", loginUser);
return "redirect:/home";
} else {
model.addAttribute("msg", "用户名或密码错误!");
return "login";
}
}
```
3. 发表个人日志功能
```
@RequestMapping(value = "/addBlog", method = RequestMethod.POST)
public String addBlog(Blog blog, HttpSession session) {
User user = (User) session.getAttribute("user");
blog.setUserId(user.getId());
blogService.addBlog(blog);
return "redirect:/home";
}
```
4. 查看个人日志和评论功能
```
@RequestMapping(value = "/viewBlog/{id}", method = RequestMethod.GET)
public String viewBlog(@PathVariable("id") Long id, Model model) {
Blog blog = blogService.getBlogById(id);
List<Comment> comments = commentService.getCommentsByBlogId(id);
model.addAttribute("blog", blog);
model.addAttribute("comments", comments);
return "viewBlog";
}
```
5. 评论个人日志和删除评论功能
```
@RequestMapping(value = "/addComment", method = RequestMethod.POST)
public String addComment(Comment comment, HttpSession session) {
User user = (User) session.getAttribute("user");
comment.setUserId(user.getId());
commentService.addComment(comment);
return "redirect:/viewBlog/" + comment.getBlogId();
}
@RequestMapping(value = "/deleteComment/{id}", method = RequestMethod.GET)
public String deleteComment(@PathVariable("id") Long id) {
Comment comment = commentService.getCommentById(id);
commentService.deleteComment(id);
return "redirect:/viewBlog/" + comment.getBlogId();
}
```
6. 删除和修改个人日志功能
```
@RequestMapping(value = "/deleteBlog/{id}", method = RequestMethod.GET)
public String deleteBlog(@PathVariable("id") Long id) {
blogService.deleteBlog(id);
commentService.deleteCommentsByBlogId(id);
return "redirect:/home";
}
@RequestMapping(value = "/editBlog/{id}", method = RequestMethod.GET)
public String editBlog(@PathVariable("id") Long id, Model model) {
Blog blog = blogService.getBlogById(id);
model.addAttribute("blog", blog);
return "editBlog";
}
@RequestMapping(value = "/updateBlog", method = RequestMethod.POST)
public String updateBlog(Blog blog) {
blogService.updateBlog(blog);
return "redirect:/home";
}
```
以上代码仅供参考,具体实现过程可能还需要考虑其他因素。
阅读全文
相关推荐
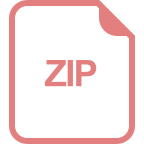
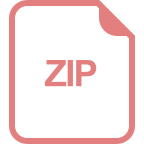
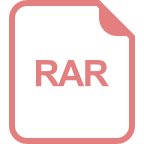
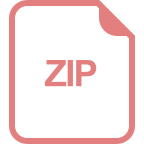
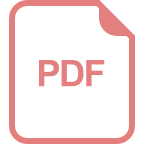
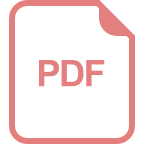
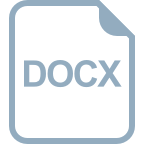
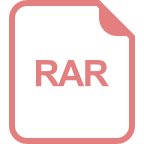
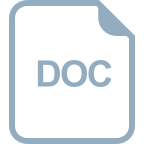
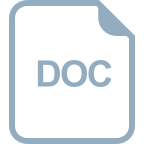
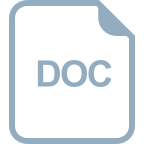
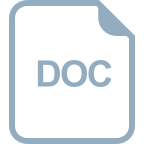
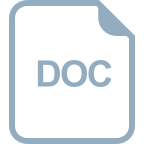
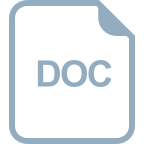
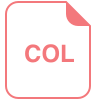
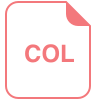
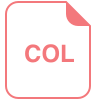
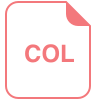