c语言线程互斥锁的使用
时间: 2024-06-11 08:09:42 浏览: 103
在C语言中,线程互斥锁是一种同步机制,用于协调多个线程之间的访问共享资源。在多线程程序中,如果多个线程同时访问同一个共享资源,会导致数据竞争和不确定性结果。为了避免这种情况,我们需要使用互斥锁来确保在任何给定时刻只有一个线程可以访问共享资源。
以下是使用C语言线程互斥锁的基本步骤:
1.包含头文件:需要包含头文件< pthread.h>,该头文件中包含了互斥锁相关的函数定义。
2.定义互斥锁:使用 pthread_mutex_t 数据类型定义互斥锁。
3.初始化互斥锁:使用 pthread_mutex_init() 函数初始化互斥锁。
4.加锁:使用 pthread_mutex_lock() 函数加锁,确保只有一个线程可以访问临界区。
5.解锁:使用 pthread_mutex_unlock() 函数解锁,允许其他线程访问临界区。
6.销毁互斥锁:使用 pthread_mutex_destroy() 函数销毁互斥锁。
下面是一个简单的示例程序,展示了如何使用互斥锁来保护共享变量:
```c
#include <pthread.h>
#include <stdio.h>
pthread_mutex_t mutex;
int count = 0;
void* increment(void* arg) {
int i;
for (i = 0; i < 10000; i++) {
pthread_mutex_lock(&mutex);
count++;
pthread_mutex_unlock(&mutex);
}
return NULL;
}
int main() {
pthread_t thread1, thread2;
// 初始化互斥锁
pthread_mutex_init(&mutex, NULL);
// 创建线程
pthread_create(&thread1, NULL, increment, NULL);
pthread_create(&thread2, NULL, increment, NULL);
// 等待线程结束
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
// 销毁互斥锁
pthread_mutex_destroy(&mutex);
printf("Count: %d\n", count);
return 0;
}
```
在上面的示例程序中,我们定义了一个名为 count 的共享变量,并创建了两个线程来对其进行加法操作。在每个线程的循环中,我们使用 pthread_mutex_lock() 函数来锁定互斥锁,以确保每个线程都可以独立访问临界区。在 count 变量更新后,我们使用 pthread_mutex_unlock() 函数来解锁互斥锁,允许其他线程访问临界区。最后,我们使用 pthread_mutex_destroy() 函数销毁互斥锁,并输出最终的 count 值。
通过使用互斥锁,我们可以确保多个线程之间的共享资源访问是安全和有序的。
阅读全文
相关推荐
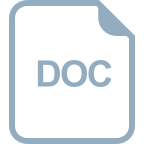
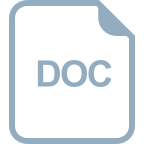


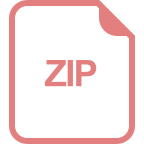
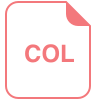
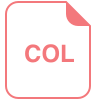
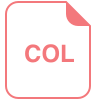
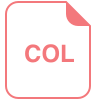






