首页如何将浏览器抓包请求代码变成python格式
如何将浏览器抓包请求代码变成python格式
时间: 2023-02-08 07:09:38 浏览: 53
可以使用 Python 的 requests 库来模拟浏览器发出的请求。通过使用 requests.get() 和 requests.post() 方法,可以发送 GET 和 POST 请求。还可以通过设置 headers 参数来模拟浏览器的请求头。
示例代码:
```python
import requests
headers = {'user-agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/89.0.4389.82 Safari/537.36'}
response = requests.get("http://www.example.com", headers=headers)
print(response.content)
```
这段代码使用了带有浏览器 user-agent 的请求头,发出了对 "http://www.example.com" 的 GET 请求,并打印出响应内容。
通过模拟浏览器请求并解析响应,就可以使用 Python 自动化网络抓包了。
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
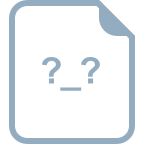
node-v12.22.1-linux-x64.tar.xz
Node.js,简称Node,是一个开源且跨平台的JavaScript运行时环境,它允许在浏览器外运行JavaScript代码。Node.js于2009年由Ryan Dahl创立,旨在创建高性能的Web服务器和网络应用程序。它基于Google Chrome的V8 JavaScript引擎,可以在Windows、Linux、Unix、Mac OS X等操作系统上运行。
Node.js的特点之一是事件驱动和非阻塞I/O模型,这使得它非常适合处理大量并发连接,从而在构建实时应用程序如在线游戏、聊天应用以及实时通讯服务时表现卓越。此外,Node.js使用了模块化的架构,通过npm(Node package manager,Node包管理器),社区成员可以共享和复用代码,极大地促进了Node.js生态系统的发展和扩张。
Node.js不仅用于服务器端开发。随着技术的发展,它也被用于构建工具链、开发桌面应用程序、物联网设备等。Node.js能够处理文件系统、操作数据库、处理网络请求等,因此,开发者可以用JavaScript编写全栈应用程序,这一点大大提高了开发效率和便捷性。
在实践中,许多大型企业和组织已经采用Node.js作为其Web应用程序的开发平台,如Netflix、PayPal和Walmart等。它们利用Node.js提高了应用性能,简化了开发流程,并且能更快地响应市场需求。
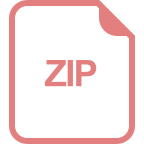
基于微信小程序的校园综合服务小程序
大学生毕业设计、大学生课程设计作业
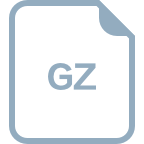
node-v7.8.0-linux-armv7l.tar.gz
Node.js,简称Node,是一个开源且跨平台的JavaScript运行时环境,它允许在浏览器外运行JavaScript代码。Node.js于2009年由Ryan Dahl创立,旨在创建高性能的Web服务器和网络应用程序。它基于Google Chrome的V8 JavaScript引擎,可以在Windows、Linux、Unix、Mac OS X等操作系统上运行。
Node.js的特点之一是事件驱动和非阻塞I/O模型,这使得它非常适合处理大量并发连接,从而在构建实时应用程序如在线游戏、聊天应用以及实时通讯服务时表现卓越。此外,Node.js使用了模块化的架构,通过npm(Node package manager,Node包管理器),社区成员可以共享和复用代码,极大地促进了Node.js生态系统的发展和扩张。
Node.js不仅用于服务器端开发。随着技术的发展,它也被用于构建工具链、开发桌面应用程序、物联网设备等。Node.js能够处理文件系统、操作数据库、处理网络请求等,因此,开发者可以用JavaScript编写全栈应用程序,这一点大大提高了开发效率和便捷性。
在实践中,许多大型企业和组织已经采用Node.js作为其Web应用程序的开发平台,如Netflix、PayPal和Walmart等。它们利用Node.js提高了应用性能,简化了开发流程,并且能更快地响应市场需求。
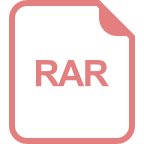
毕业设计:Python招聘分析系统论文(源码 + 数据库 + 说明文档)
毕业设计:Python招聘分析系统论文(源码 + 数据库 + 说明文档)
2 需求分析 9
2.1功能需求分析 9
2.2 可行性分析 9
2.2.1 技术可行性 9
2.2.2 经济可行性 9
2.2.3 操作可行性 10
2.2.4 发展可行性 10
2.3系统性需求分析 10
2.4招聘分析系统管理功能 11
3 总体设计 12
3.1 系统结构 12
3.2 数据库设计 12
3.2.1 数据库实体 12
3.2.2 数据库表设计 13
4 运行设计 15
4.1 招聘热门行业分析 15
4.2热门岗位分析界面 15
4.3招聘岗位学历分析界面 16
4.4岗位分布分析界面 16
5 系统测试 18
5.1测试环境与条件 18
5.2功能测试 18
5.3安全测试 18
5.4可用性测试 18
5.5测试结果分析 19
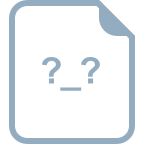
win11修复-网络正常-永远是地球小图标的bug
win11修复-网络正常-永远是地球小图标的bug
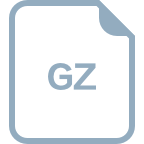
RTL8188FU-Linux-v5.7.4.2-36687.20200602.tar(20765).gz
REALTEK 8188FTV 8188eus 8188etv linux驱动程序稳定版本, 支持AP,STA 以及AP+STA 共存模式。 稳定支持linux4.0以上内核。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
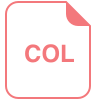
:YOLOv1目标检测算法:实时目标检测的先驱,开启计算机视觉新篇章
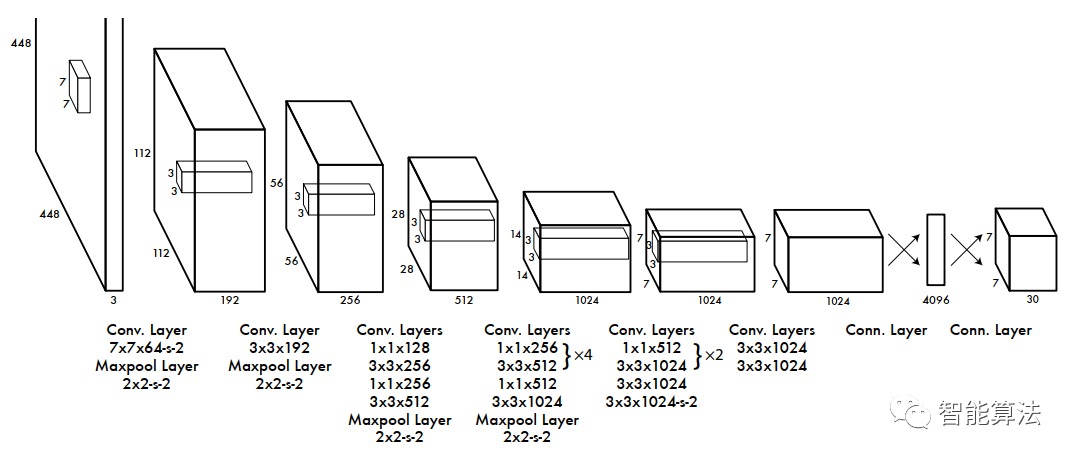
# 1. 目标检测算法概述
目标检测算法是一种计算机视觉技术,用于识别和定位图像或视频中的对象。它在各种应用中至关重要,例如自动驾驶、视频监控和医疗诊断。
目标检测算法通常分为两类:两阶段算法和单阶段算法。两阶段算法,如 R-CNN 和 Fast R-CNN,首先生成候选区域,然后对每个区域进行分类和边界框回归。单阶段算法,如 YOLO 和 SSD,一次性执行检

设计算法实现将单链表中数据逆置后输出。用C语言代码
如下所示:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义单链表节点结构体
struct node {
int data;
struct node *next;
};
// 定义单链表逆置函数
struct node* reverse(struct node *head) {
struct node *prev = NULL;
struct node *curr = head;
struct node *next;
while (curr != NULL) {
next
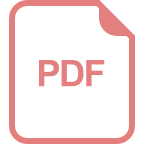
c++校园超市商品信息管理系统课程设计说明书(含源代码) (2).pdf
校园超市商品信息管理系统课程设计旨在帮助学生深入理解程序设计的基础知识,同时锻炼他们的实际操作能力。通过设计和实现一个校园超市商品信息管理系统,学生掌握了如何利用计算机科学与技术知识解决实际问题的能力。在课程设计过程中,学生需要对超市商品和销售员的关系进行有效管理,使系统功能更全面、实用,从而提高用户体验和便利性。
学生在课程设计过程中展现了积极的学习态度和纪律,没有缺勤情况,演示过程流畅且作品具有很强的使用价值。设计报告完整详细,展现了对问题的深入思考和解决能力。在答辩环节中,学生能够自信地回答问题,展示出扎实的专业知识和逻辑思维能力。教师对学生的表现予以肯定,认为学生在课程设计中表现出色,值得称赞。
整个课程设计过程包括平时成绩、报告成绩和演示与答辩成绩三个部分,其中平时表现占比20%,报告成绩占比40%,演示与答辩成绩占比40%。通过这三个部分的综合评定,最终为学生总成绩提供参考。总评分以百分制计算,全面评估学生在课程设计中的各项表现,最终为学生提供综合评价和反馈意见。
通过校园超市商品信息管理系统课程设计,学生不仅提升了对程序设计基础知识的理解与应用能力,同时也增强了团队协作和沟通能力。这一过程旨在培养学生综合运用技术解决问题的能力,为其未来的专业发展打下坚实基础。学生在进行校园超市商品信息管理系统课程设计过程中,不仅获得了理论知识的提升,同时也锻炼了实践能力和创新思维,为其未来的职业发展奠定了坚实基础。
校园超市商品信息管理系统课程设计的目的在于促进学生对程序设计基础知识的深入理解与掌握,同时培养学生解决实际问题的能力。通过对系统功能和用户需求的全面考量,学生设计了一个实用、高效的校园超市商品信息管理系统,为用户提供了更便捷、更高效的管理和使用体验。
综上所述,校园超市商品信息管理系统课程设计是一项旨在提升学生综合能力和实践技能的重要教学活动。通过此次设计,学生不仅深化了对程序设计基础知识的理解,还培养了解决实际问题的能力和团队合作精神。这一过程将为学生未来的专业发展提供坚实基础,使其在实际工作中能够胜任更多挑战。