字符串类定义输入字符串函数
时间: 2023-06-16 09:06:39 浏览: 107
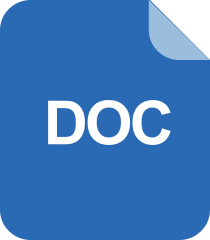
字符串操作函数
以下是一个示例的字符串类定义,其中包含一个输入字符串的函数:
```c++
#include <iostream>
#include <cstring>
class String {
private:
char* m_data; // 字符串数据
size_t m_size; // 字符串长度
public:
// 默认构造函数
String() : m_data(nullptr), m_size(0) {}
// 构造函数
String(const char* str) : m_data(nullptr), m_size(0) {
if (str != nullptr) {
m_size = strlen(str);
m_data = new char[m_size + 1];
strcpy(m_data, str);
}
}
// 拷贝构造函数
String(const String& other) : m_data(nullptr), m_size(other.m_size) {
if (other.m_data != nullptr) {
m_data = new char[m_size + 1];
strcpy(m_data, other.m_data);
}
}
// 析构函数
~String() {
if (m_data != nullptr) {
delete[] m_data;
m_data = nullptr;
m_size = 0;
}
}
// 重载赋值运算符
String& operator=(const String& other) {
if (this != &other) {
String tmp(other);
std::swap(m_data, tmp.m_data);
std::swap(m_size, tmp.m_size);
}
return *this;
}
// 输入字符串函数
void input() {
char buf[1024];
std::cin.getline(buf, 1024);
String tmp(buf);
*this = tmp;
}
// 获取字符串长度
size_t size() const {
return m_size;
}
// 获取字符串数据
const char* data() const {
return m_data;
}
};
```
这个字符串类定义包含一个 `input()` 函数,可以从标准输入流中读取一行字符串,并将其赋值给当前字符串对象。在这个函数中,我们使用 `std::cin.getline()` 函数读取字符串,并将其存储在一个临时的 `String` 对象中,然后通过重载赋值运算符将其赋值给当前对象。
使用这个字符串类,可以像下面这样输入字符串:
```c++
int main() {
String str;
std::cout << "Please input a string: ";
str.input();
std::cout << "The input string is: " << str.data() << std::endl;
return 0;
}
```
阅读全文
相关推荐
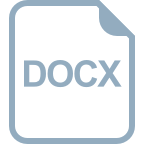
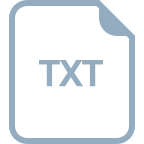
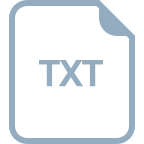
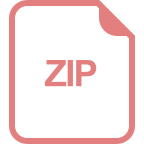
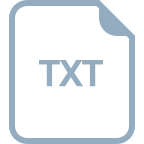
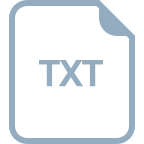
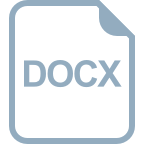
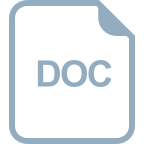
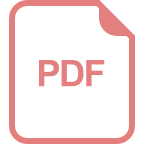
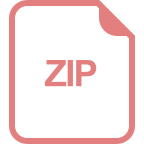
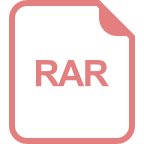
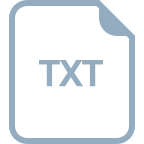
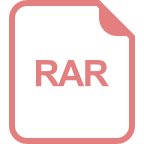