java 写一个快速排序
时间: 2023-09-20 09:05:56 浏览: 43
### 回答1:
好的,下面给出Java实现的快速排序代码:
```
public class QuickSort {
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pivotIndex = partition(arr, low, high);
quickSort(arr, low, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, high);
}
}
private static int partition(int[] arr, int low, int high) {
int pivot = arr[high];
int i = low - 1;
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
swap(arr, i, j);
}
}
swap(arr, i + 1, high);
return i + 1;
}
private static void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
```
使用方法:
```
int[] arr = {4, 3, 6, 1, 2, 5};
QuickSort.quickSort(arr, 0, arr.length - 1);
System.out.println(Arrays.toString(arr));
```
输出结果为:[1, 2, 3, 4, 5, 6]
### 回答2:
快速排序是一种经典的排序算法,它的时间复杂度为 O(nlogn)。下面是用 Java 语言实现快速排序的代码:
```java
public class QuickSort {
public static void main(String[] args) {
int[] array = {9, 2, 5, 7, 1, 3, 8, 6, 4};
quickSort(array, 0, array.length - 1);
for (int num : array) {
System.out.print(num + " ");
}
}
public static void quickSort(int[] array, int start, int end) {
if (start < end) {
int pivotIndex = partition(array, start, end);
quickSort(array, start, pivotIndex - 1);
quickSort(array, pivotIndex + 1, end);
}
}
public static int partition(int[] array, int start, int end) {
int pivot = array[end];
int i = start - 1;
for (int j = start; j < end; j++) {
if (array[j] < pivot) {
i++;
// 交换 array[i] 和 array[j]
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
// 交换 array[i+1] 和 array[end]
int temp = array[i + 1];
array[i + 1] = array[end];
array[end] = temp;
return i + 1;
}
}
```
以上代码中,`quickSort` 方法实现了快速排序算法,`partition` 方法用来将数组分区。在 `quickSort` 方法中,首先选取一个基准元素(数组最后一个元素),将小于基准的元素放在基准的左边,大于基准的元素放在基准的右边,然后分别对左右两个子数组进行递归排序。最后打印排序后的数组结果。
### 回答3:
快速排序是一种常见的排序算法,它基于分而治之的思想。下面是用Java编写的一个快速排序算法的示例:
```java
public class QuickSort {
public static void quickSort(int[] arr, int start, int end) {
if (start < end) {
int pivotIndex = partition(arr, start, end);
quickSort(arr, start, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, end);
}
}
private static int partition(int[] arr, int start, int end) {
int pivot = arr[end];
int i = start - 1;
for (int j = start; j < end; j++) {
if (arr[j] <= pivot) {
i++;
swap(arr, i, j);
}
}
swap(arr, i + 1, end);
return i + 1;
}
private static void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
public static void main(String[] args) {
int[] arr = {5, 3, 8, 4, 2};
quickSort(arr, 0, arr.length - 1);
System.out.println("排序后的数组:");
for (int num : arr) {
System.out.print(num + " ");
}
}
}
```
快速排序的原理是选择一个基准元素(通常为数组中的最后一个元素),将数组划分为两个子数组,一个小于等于基准元素,另一个大于基准元素。然后对这两个子数组分别进行递归调用快速排序算法,将子数组排序。
以上示例中,`quickSort`方法用于调用快速排序算法,`partition`方法用于划分子数组,并返回基准元素的最终索引。`swap`方法用于交换数组中的两个元素。`main`方法用于测试排序结果,并打印排序后的数组。
运行以上程序,输出结果为:2 3 4 5 8,表示按照从小到大的顺序对数组进行了排序。
相关推荐



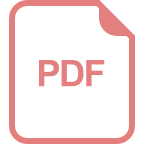
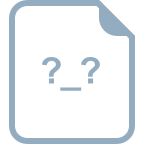
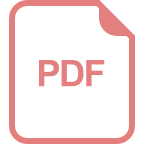