用代码生成一个图书馆管理系统
时间: 2023-09-13 19:05:32 浏览: 124
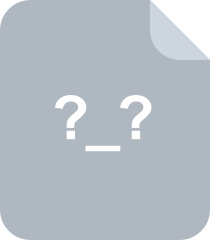
图书馆管理系统的代码
好的,以下是一个简单的图书馆管理系统的 Python 代码示例:
```python
class Book:
def __init__(self, title, author, ISBN, copies):
self.title = title
self.author = author
self.ISBN = ISBN
self.copies = copies
class Library:
def __init__(self):
self.books = []
def add_book(self, book):
self.books.append(book)
def remove_book(self, book):
self.books.remove(book)
def search_book(self, title):
for book in self.books:
if book.title == title:
return book
return None
def checkout_book(self, title):
book = self.search_book(title)
if book is not None and book.copies > 0:
book.copies -= 1
return True
return False
def checkin_book(self, title):
book = self.search_book(title)
if book is not None:
book.copies += 1
return True
return False
library = Library()
book1 = Book("Harry Potter and the Philosopher's Stone", "J.K. Rowling", "9780747532743", 5)
book2 = Book("The Lord of the Rings", "J.R.R. Tolkien", "9780544003415", 3)
library.add_book(book1)
library.add_book(book2)
while True:
print("1. Search for a book")
print("2. Checkout a book")
print("3. Checkin a book")
print("4. Exit")
choice = input("Enter your choice: ")
if choice == "1":
title = input("Enter the title of the book: ")
book = library.search_book(title)
if book is not None:
print("Title:", book.title)
print("Author:", book.author)
print("ISBN:", book.ISBN)
print("Copies available:", book.copies)
else:
print("Book not found")
elif choice == "2":
title = input("Enter the title of the book: ")
if library.checkout_book(title):
print("Book checked out successfully")
else:
print("Book not available")
elif choice == "3":
title = input("Enter the title of the book: ")
if library.checkin_book(title):
print("Book checked in successfully")
else:
print("Book not found")
elif choice == "4":
break
else:
print("Invalid choice")
```
这个系统包括两个类:`Book` 类表示一本书,包括标题、作者、ISBN 号和副本数等属性;`Library` 类表示图书馆,包括一个可以添加、删除、搜索、借阅和归还书籍的方法。在 `Library` 类的构造函数中,我们初始化一个空的书籍列表。在 `while` 循环中,我们提供了四个选项:搜索书籍、借阅书籍、归还书籍和退出系统。用户可以根据需要选择适当的选项。
阅读全文
相关推荐
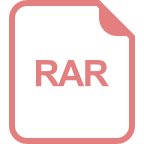
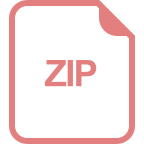
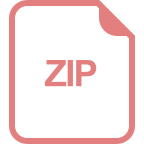
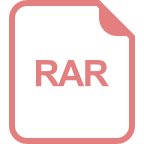
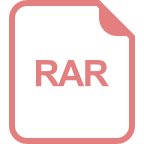
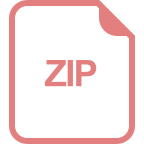
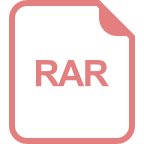
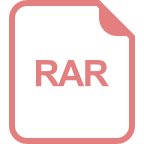
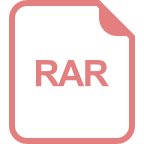
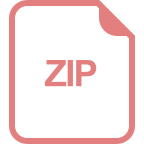
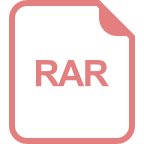
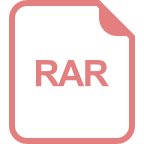
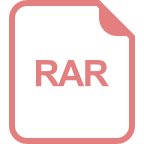