数据库有60w条数据,如何使用多线程对这些数据进行修改,具体实现
时间: 2024-03-17 13:40:54 浏览: 44
具体实现流程如下:
1. 将数据库的数据分成多个批次,每个批次包含一定数量的数据。
2. 创建多个线程,每个线程负责处理一个批次的数据。
3. 在每个线程中连接数据库,查询需要修改的数据,然后进行修改操作。
4. 在进行修改操作时,要注意数据的一致性和并发性,可以使用数据库锁机制或者乐观锁机制来保证数据的正确性。
5. 修改完成后,提交事务并关闭数据库连接。
6. 当所有线程处理完成后,合并数据。
下面是一个简单的示例代码:
```python
import threading
import pymysql
# 数据库连接信息
db_config = {
'host': 'localhost',
'port': 3306,
'user': 'root',
'password': '123456',
'database': 'test'
}
# 每个批次包含的数据量
batch_size = 1000
# 修改数据的 SQL 语句
update_sql = 'UPDATE user SET age = age + 1 WHERE id = %s'
# 查询数据的 SQL 语句
select_sql = 'SELECT id FROM user LIMIT %s, %s'
def process_data(start, end):
# 连接数据库
conn = pymysql.connect(**db_config)
cursor = conn.cursor()
# 查询需要修改的数据
cursor.execute(select_sql, (start, batch_size))
results = cursor.fetchall()
# 修改数据
for result in results:
cursor.execute(update_sql, (result[0],))
# 提交事务并关闭数据库连接
conn.commit()
cursor.close()
conn.close()
if __name__ == '__main__':
# 计算需要分成的批次数量
total_count = 600000
batch_count = total_count // batch_size + 1
# 创建多个线程
threads = []
for i in range(batch_count):
start = i * batch_size
end = start + batch_size
if end > total_count:
end = total_count
thread = threading.Thread(target=process_data, args=(start, end))
threads.append(thread)
thread.start()
# 等待所有线程执行完成
for thread in threads:
thread.join()
# 合并数据
# ...
```
需要注意的是,这只是一个简单的示例代码,实际应用中还需要考虑一些细节问题,如线程安全、错误处理、数据合并等。
阅读全文
相关推荐


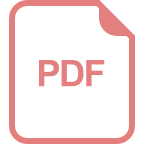
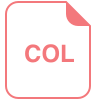
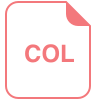
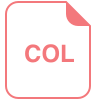
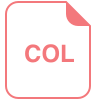
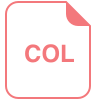
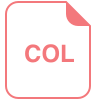
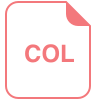
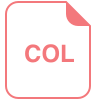
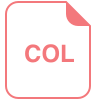
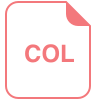
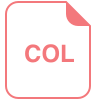
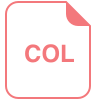