编写一段Java代码,使用gui界面输出实现简单算术表示式到抽象语法树的转换。具体要求如下输入以#结束的中缀表达式(包括+-*/()数字),例如:x=a+b*c;
时间: 2024-02-01 09:16:00 浏览: 131
以下是一个简单的Java代码,使用GUI界面输出实现算术表达式到抽象语法树的转换:
```java
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.Font;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Stack;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.SwingConstants;
import javax.swing.border.EmptyBorder;
public class ExpressionTreeGUI extends JFrame {
private JPanel contentPane;
private JTextField textField;
private JLabel resultLabel;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
ExpressionTreeGUI frame = new ExpressionTreeGUI();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public ExpressionTreeGUI() {
setTitle("Expression Tree Converter");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 450, 300);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
JPanel inputPanel = new JPanel();
contentPane.add(inputPanel, BorderLayout.NORTH);
JLabel inputLabel = new JLabel("Input Infix Expression:");
inputPanel.add(inputLabel);
textField = new JTextField();
inputPanel.add(textField);
textField.setColumns(20);
JButton convertButton = new JButton("Convert");
convertButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String infix = textField.getText();
String postfix = infixToPostfix(infix);
TreeNode root = postfixToTree(postfix);
resultLabel.setText("Result: " + root.toString());
}
});
inputPanel.add(convertButton);
JPanel outputPanel = new JPanel();
contentPane.add(outputPanel, BorderLayout.CENTER);
outputPanel.setLayout(new GridLayout(0, 1, 0, 0));
resultLabel = new JLabel("Result:");
resultLabel.setFont(new Font("Lucida Grande", Font.BOLD, 16));
resultLabel.setHorizontalAlignment(SwingConstants.CENTER);
outputPanel.add(resultLabel);
}
private int precedence(char operator) {
if (operator == '+' || operator == '-') {
return 1;
} else if (operator == '*' || operator == '/') {
return 2;
} else {
return 0;
}
}
private String infixToPostfix(String infix) {
StringBuilder postfix = new StringBuilder();
Stack<Character> stack = new Stack<>();
for (int i = 0; i < infix.length(); i++) {
char ch = infix.charAt(i);
if (ch == ' ') {
continue;
}
if (Character.isDigit(ch)) {
postfix.append(ch);
} else if (ch == '+' || ch == '-' || ch == '*' || ch == '/') {
while (!stack.empty() && precedence(ch) <= precedence(stack.peek())) {
postfix.append(stack.pop());
}
stack.push(ch);
} else if (ch == '(') {
stack.push(ch);
} else if (ch == ')') {
while (!stack.empty() && stack.peek() != '(') {
postfix.append(stack.pop());
}
stack.pop();
}
}
while (!stack.empty()) {
postfix.append(stack.pop());
}
return postfix.toString();
}
private TreeNode postfixToTree(String postfix) {
Stack<TreeNode> stack = new Stack<>();
for (int i = 0; i < postfix.length(); i++) {
char ch = postfix.charAt(i);
if (Character.isDigit(ch)) {
TreeNode node = new TreeNode(ch - '0');
stack.push(node);
} else {
TreeNode right = stack.pop();
TreeNode left = stack.pop();
TreeNode node = new TreeNode(ch, left, right);
stack.push(node);
}
}
return stack.pop();
}
private class TreeNode {
char operator;
int operand;
TreeNode left;
TreeNode right;
public TreeNode(char operator, TreeNode left, TreeNode right) {
this.operator = operator;
this.left = left;
this.right = right;
}
public TreeNode(int operand) {
this.operand = operand;
}
@Override
public String toString() {
if (operand != 0) {
return Integer.toString(operand);
} else {
String leftStr = left.toString();
String rightStr = right.toString();
return "(" + leftStr + " " + operator + " " + rightStr + ")";
}
}
}
}
```
这个程序使用了Swing库来创建GUI界面。用户可以输入一个中缀表达式,然后单击“Convert”按钮,程序将把该表达式转换为后缀表达式,然后构建一个抽象语法树,并将其显示在GUI中。程序使用了一个栈来实现转换和构建树的过程。
阅读全文
相关推荐







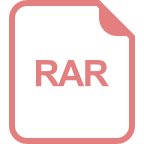



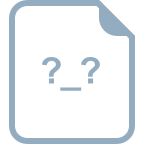




