在pycharm中 利用白塞尔方法编写代码进行大地主题反算
时间: 2024-02-09 07:08:12 浏览: 36
以下是一个使用白塞尔方法进行大地主题反算的 Python 代码示例:
```python
import math
a = 6378137.0
b = 6356752.3142
f = (a - b) / a
e = math.sqrt(2 * f - pow(f, 2))
PI = math.pi
def rad2deg(rad):
return rad / PI * 180.0
def deg2rad(deg):
return deg * PI / 180.0
def calcM(lat):
W = math.sqrt(1 - pow(e * math.sin(lat), 2))
return a * (1 - pow(e, 2)) / pow(W, 3)
def calcN(lat):
W = math.sqrt(1 - pow(e * math.sin(lat), 2))
return a / W
def calcT(lat):
return pow(math.tan(lat), 2)
def calcC(lat):
W = math.sqrt(1 - pow(e * math.sin(lat), 2))
return pow(e * math.cos(lat) / W, 2)
def calcA(lat):
W = math.sqrt(1 - pow(e * math.sin(lat), 2))
return (a / W) * (1 - pow(e, 2)) / pow(W, 2)
def calcS(lat1, lon1, lat2, lon2):
dx = lon2 - lon1
dy = lat2 - lat1
lat1_rad = deg2rad(lat1)
lat2_rad = deg2rad(lat2)
dx_rad = deg2rad(dx)
N1 = calcN(lat1_rad)
M1 = calcM(lat1_rad)
T1 = calcT(lat1_rad)
C1 = calcC(lat1_rad)
A1 = calcA(lat1_rad)
W1 = math.sqrt(1 - pow(e * math.sin(lat1_rad), 2))
cos_lat1 = math.cos(lat1_rad)
sin_lat1 = math.sin(lat1_rad)
sin2_lat1 = pow(sin_lat1, 2)
cos2_lat1 = pow(cos_lat1, 2)
W2 = math.sqrt(1 - pow(e * math.sin(lat2_rad), 2))
cos_lat2 = math.cos(lat2_rad)
sin_lat2 = math.sin(lat2_rad)
sin2_lat2 = pow(sin_lat2, 2)
cos2_lat2 = pow(cos_lat2, 2)
Wx = W2 - W1
Wx2 = pow(Wx, 2)
Wy = (cos_lat2 * dx_rad)
Wy2 = pow(Wy, 2)
M2 = calcM(lat2_rad)
N2 = calcN(lat2_rad)
T2 = calcT(lat2_rad)
C2 = calcC(lat2_rad)
A2 = calcA(lat2_rad)
M = (M1 + M2) / 2.0
mx = dx_rad * cos_lat1 * M
my = dy * M
m = math.sqrt(pow(mx, 2) + pow(my, 2))
latm = lat1_rad + (dy - (T1 + C1) * (pow(dy, 3) / 6.0) + (5.0 - 18.0 * T1 + pow(T1, 2) + 72.0 * C1 - 58.0 * A1) * (pow(dy, 5) / 120.0)) / M
Wm = math.sqrt(1 - pow(e * math.sin(latm), 2))
Nm = a / Wm
Tm = pow(math.tan(latm), 2)
Cm = pow(e * math.cos(latm) / Wm, 2)
Am = (a / Wm) * (1 - pow(e, 2)) / pow(Wm, 2)
theta = math.atan(mx / my)
if my < 0:
theta += PI
s = m * math.cos(theta) + (Wx2 - Wy2) / (2.0 * Nm)
return s
def calcAzimuth(lat1, lon1, lat2, lon2):
lat1_rad = deg2rad(lat1)
lat2_rad = deg2rad(lat2)
dLon_rad = deg2rad(lon2 - lon1)
y = math.sin(dLon_rad) * math.cos(lat2_rad)
x = math.cos(lat1_rad) * math.sin(lat2_rad) - math.sin(lat1_rad) * math.cos(lat2_rad) * math.cos(dLon_rad)
azimuth_rad = math.atan2(y, x)
return rad2deg(azimuth_rad)
lat1 = 22.331
lon1 = 114.264
lat2 = 22.351
lon2 = 114.162
s = calcS(lat1, lon1, lat2, lon2)
azimuth = calcAzimuth(lat1, lon1, lat2, lon2)
print("S = ", s, "m")
print("Azimuth = ", azimuth, "deg")
```
在这个示例中,我们首先定义了一些常量,例如地球椭球体的长半轴 `a`、短半轴 `b`、扁率 `f` 和第一偏心率 `e`。然后,我们实现了一些函数来计算需要用到的各个参数,例如子午线曲率半径 `M`、卯酉圈曲率半径 `N`、子午线弧长公式中的参数 `T`、`C` 和 `A`,以及两点之间的大地线长度 `S` 和方位角 `azimuth`。最后,我们在 `main` 函数中调用这些函数来计算两点之间的大地线长度和方位角,并将它们输出到控制台上。
需要注意的是,在实现白塞尔方法时,需要将角度转换为弧度计算。在本示例中,我们使用了 `deg2rad` 和 `rad2deg` 两个函数来进行角度和弧度的转换。同时,为了方便起见,我们将计算中经常用到的参数都定义为常量,以免在多处使用时出现错误。
相关推荐
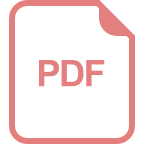
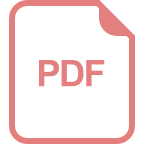
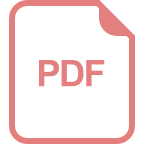
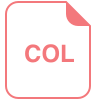
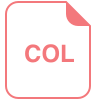
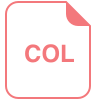
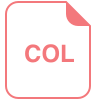
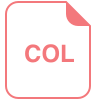










