用python写一个神经网络算法
时间: 2024-05-12 15:21:12 浏览: 63
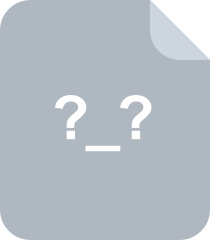
python写神经网络
以下是一个简单的神经网络算法实现:
1.导入所需的库
```
import numpy as np
```
2.定义sigmoid函数
```
def sigmoid(x):
return 1/(1+np.exp(-x))
```
3.定义神经网络类
```
class NeuralNetwork:
def __init__(self, x, y):
self.input = x
self.weights1 = np.random.rand(self.input.shape[1],4)
self.weights2 = np.random.rand(4,1)
self.y = y
self.output = np.zeros(self.y.shape)
def feedforward(self):
self.layer1 = sigmoid(np.dot(self.input, self.weights1))
self.output = sigmoid(np.dot(self.layer1, self.weights2))
def backprop(self):
d_weights2 = np.dot(self.layer1.T, (2*(self.y - self.output) * sigmoid_derivative(self.output)))
d_weights1 = np.dot(self.input.T, (np.dot(2*(self.y - self.output) * sigmoid_derivative(self.output), self.weights2.T) * sigmoid_derivative(self.layer1)))
self.weights1 += d_weights1
self.weights2 += d_weights2
```
4.训练神经网络
```
X = np.array([[0,0,1],[0,1,1],[1,0,1],[1,1,1]])
y = np.array([[0],[1],[1],[0]])
nn = NeuralNetwork(X,y)
for i in range(1500):
nn.feedforward()
nn.backprop()
print(nn.output)
```
这个神经网络算法使用了一个输入层、一个隐层和一个输出层,其中隐层有4个神经元。在训练过程中,我们使用反向传播算法来更新神经网络的权重。最终输出的结果是一个4x1的矩阵,表示对应每个输入的预测结果。
阅读全文
相关推荐
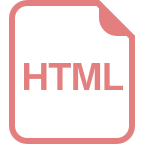
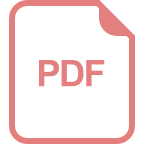


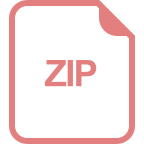
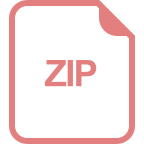
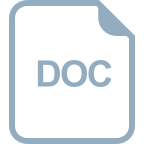
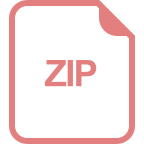
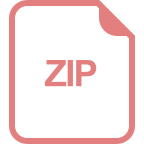
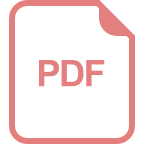
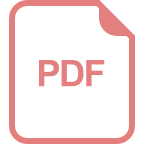
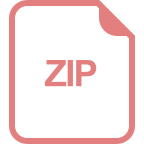


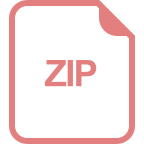