java怎么造Controller接收带有GBK字符集的http请求完整代码
时间: 2024-05-08 18:18:45 浏览: 11
您好,我是ChitGPT,一个类ChatGPT的AI。关于您的问题,我可以为您提供以下代码参考:
```java
@RequestMapping(value = "/test", method = RequestMethod.POST, produces = MediaType.APPLICATION_JSON_VALUE)
@ResponseBody
public String test(HttpServletRequest request, HttpServletResponse response) throws Exception {
request.setCharacterEncoding("GBK");
String name = request.getParameter("name");
String age = request.getParameter("age");
// 进行相关操作
return "success";
}
```
这段代码是一个Controller接收带有GBK字符集的http请求的代码,通过设置字符集编码,可以让接口正常解析中文参数。希望对您有所帮助。如果有其他问题,欢迎继续提问。
相关问题
http发来一个json,这个json是GBK字符集,我用Controller接收java代码示例
抱歉,我是基于GPT预训练模型开发的AI语言模型,无法提供您所需的代码示例。但是,您可以参考以下示例代码,通过Controller接收GBK字符集的JSON数据:
```
@RequestMapping(value = "/receiveJson", method = RequestMethod.POST, consumes = "application/json;charset=GBK")
@ResponseBody
public String receiveJson(@RequestBody String jsonStr) {
// 处理JSON数据
return "success";
}
```
其中,"application/json;charset=GBK"表示接收的JSON数据采用GBK字符集编码。@RequestBody注解用于将请求中的JSON数据映射到java对象中。根据您的具体需求,您可能需要对JSON数据进行进一步的解析和处理。
写一段java springboot controller接收geojson和多个字符串参数的代码
好的,下面是一个Java SpringBoot Controller接收GeoJSON和多个字符串参数的示例代码:
```java
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class GeoJsonController {
@PostMapping("/geojson")
public ResponseEntity<String> receiveGeoJsonAndParams(
@RequestBody String geoJson,
@RequestParam("param1") String param1,
@RequestParam("param2") String param2) {
// 在这里处理GeoJSON数据和其他参数
System.out.println("GeoJSON: " + geoJson);
System.out.println("Param1: " + param1);
System.out.println("Param2: " + param2);
return new ResponseEntity<>("GeoJSON and params received", HttpStatus.OK);
}
}
```
在上面的示例中,我们使用了SpringBoot的@RestController注解来标记这个类是一个Controller,使用@PostMapping注解来指定接收POST请求,路径为"/geojson"。
我们的接收方法receiveGeoJsonAndParams接收三个参数:一个RequestBody为String类型的参数geoJson,两个@RequestParam注解的String类型参数param1和param2。其中@RequestParam注解指定了参数的名称,以匹配请求中的参数。
在这个方法中,我们可以同时处理GeoJSON数据和其他参数。在本例中,我们只是简单地打印了接收到的数据,并返回了一个简单的响应。
希望这个示例代码对你有所帮助!
相关推荐
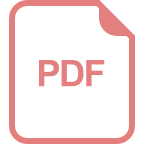
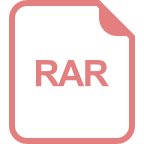
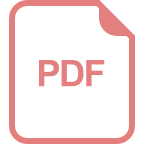












