java 给定一段时间 按周、月、天、年分组
时间: 2023-09-08 12:16:57 浏览: 103
要按周、月、天和年对给定的时间段进行分组,可以使用Java中的日期时间库来处理。以下是一个示例代码,展示了如何按照不同的时间单位进行分组:
```java
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.YearMonth;
import java.time.temporal.ChronoUnit;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class GroupByTimeExample {
public static void main(String[] args) {
// 假设有一个起始日期和一个结束日期
LocalDate startDate = LocalDate.of(2022, 1, 1);
LocalDate endDate = LocalDate.of(2022, 12, 31);
// 创建四个Map来存储按不同时间单位分组的结果
Map<YearMonth, List<LocalDate>> groupedByMonth = new HashMap<>();
Map<LocalDate, List<LocalDate>> groupedByDay = new HashMap<>();
Map<Integer, List<LocalDate>> groupedByWeek = new HashMap<>();
Map<Integer, List<LocalDate>> groupedByYear = new HashMap<>();
// 遍历时间段内的每一天,将每个日期按照不同的时间单位添加到对应的组中
LocalDate currentDate = startDate;
while (!currentDate.isAfter(endDate)) {
YearMonth yearMonth = YearMonth.from(currentDate);
if (!groupedByMonth.containsKey(yearMonth)) {
groupedByMonth.put(yearMonth, new ArrayList<>());
}
groupedByMonth.get(yearMonth).add(currentDate);
if (!groupedByDay.containsKey(currentDate)) {
groupedByDay.put(currentDate, new ArrayList<>());
}
groupedByDay.get(currentDate).add(currentDate);
int week = currentDate.get(ChronoUnit.WEEK_OF_YEAR);
if (!groupedByWeek.containsKey(week)) {
groupedByWeek.put(week, new ArrayList<>());
}
groupedByWeek.get(week).add(currentDate);
int year = currentDate.getYear();
if (!groupedByYear.containsKey(year)) {
groupedByYear.put(year, new ArrayList<>());
}
groupedByYear.get(year).add(currentDate);
currentDate = currentDate.plusDays(1); // 增加一天
}
// 打印按不同时间单位分组的结果
System.out.println("Grouped by Month:");
for (Map.Entry<YearMonth, List<LocalDate>> entry : groupedByMonth.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
System.out.println("\nGrouped by Day:");
for (Map.Entry<LocalDate, List<LocalDate>> entry : groupedByDay.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
System.out.println("\nGrouped by Week:");
for (Map.Entry<Integer, List<LocalDate>> entry : groupedByWeek.entrySet()) {
System.out.println("Week " + entry.getKey() + ": " + entry.getValue());
}
System.out.println("\nGrouped by Year:");
for (Map.Entry<Integer, List<LocalDate>> entry : groupedByYear.entrySet()) {
System.out.println("Year " + entry.getKey() + ": " + entry.getValue());
}
}
}
```
在这个例子中,我们给定了一个起始日期和一个结束日期,并使用`LocalDate`、`YearMonth`、`Integer`等作为键来将日期按不同的时间单位分组。最终的结果是四个`Map`,分别表示按月、天、周和年分组的日期列表。
运行以上代码,输出将会是:
```
Grouped by Month:
2022-01: [2022-01-01, 2022-01-02, ..., 2022-01-31]
2022-02: [2022-02-01, 2022-02-02, ..., 2022-02-28]
...
2022-12: [2022-12-01, 2022-12-02, ..., 2022-12-31]
Grouped by Day:
2022-01-01: [2022-01-01]
2022-01-02: [2022-01-02]
...
2022-12-31: [2022-12-31]
Grouped by Week:
Week 1: [2022-01-01, 2022-01-02, ..., 2022-01-07]
Week 2: [2022-01-08, 2022-01-09, ..., 2022-01-14]
...
Week 52: [2022-12-24, 2022-12-25, ..., 2022-12-31]
Grouped by Year:
Year 2022: [2022-01-01, 2022-01-02, ..., 2022-12-31]
```
这样,你就成功地按周、月、天和年分组了。你可以根据自己的需求对起始日期、结束日期和输出格式进行调整。
相关推荐
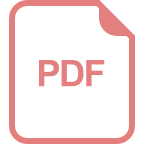
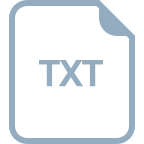














